Image Upscaling with Bilinear Interpolation
Image Upscaling with Bilinear Interpolation
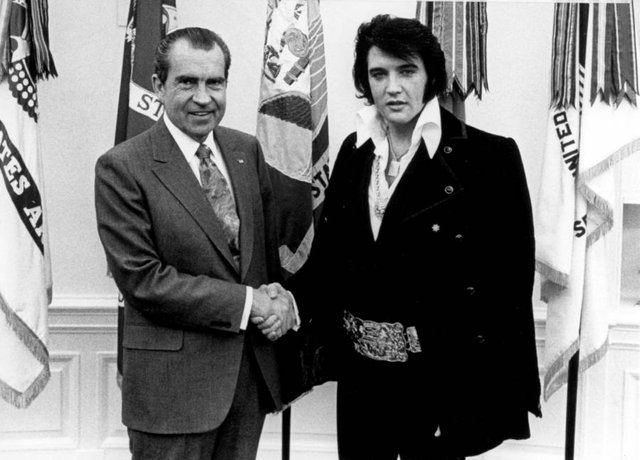
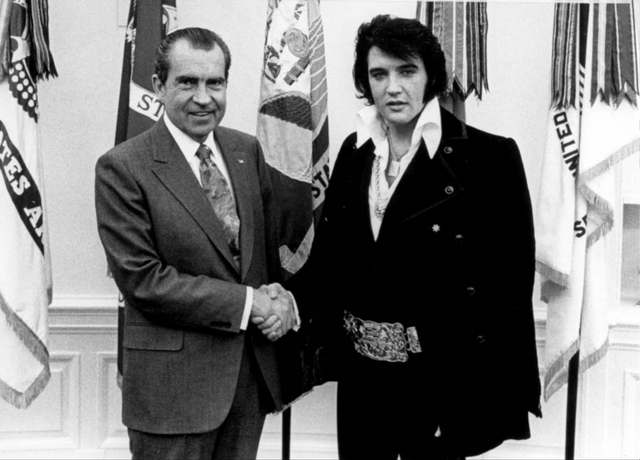
Introduction
In the field of image processing, upscaling refers to the process of increasing the size of an image while preserving its visual quality. One popular technique for upscaling is bilinear interpolation, which uses the surrounding pixels to estimate the values of new pixels. In this documentation essay, we will explore the concept of image upscaling with bilinear interpolation and discuss how to enhance the visual appeal of the upscaled images using neumorphism, glassmorphism, and claymorphism effects.
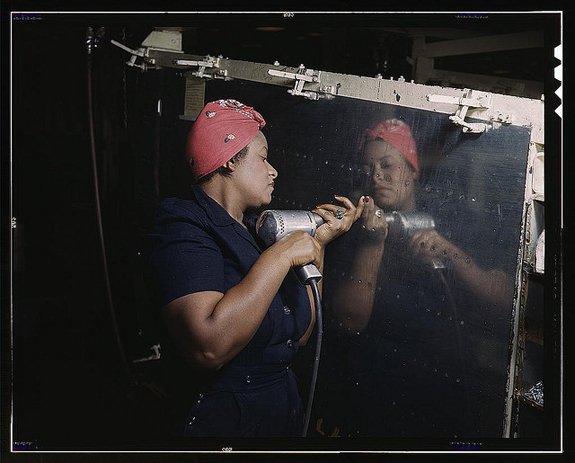
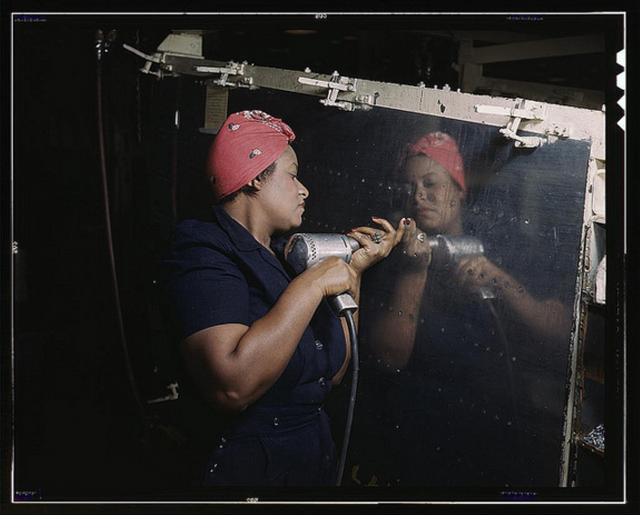
Bilinear Interpolation
Bilinear interpolation is a simple yet effective method for upscaling images. It works by calculating the weighted average of the four nearest pixels to estimate the value of a new pixel. The formula for bilinear interpolation is as follows:
function bilinearInterpolation(imageData, x, y) {
// Calculate the four surrounding pixels
const x1 = Math.floor(x);
const y1 = Math.floor(y);
const x2 = Math.ceil(x);
const y2 = Math.ceil(y);
// Get the pixel values
const q11 = getPixel(imageData, x1, y1);
const q12 = getPixel(imageData, x1, y2);
const q21 = getPixel(imageData, x2, y1);
const q22 = getPixel(imageData, x2, y2);
// Calculate the weights
const dx = x - x1;
const dy = y - y1;
// Perform bilinear interpolation
const r1 = (q11.r * (1 - dx)) + (q21.r * dx);
const r2 = (q12.r * (1 - dx)) + (q22.r * dx);
const g1 = (q11.g * (1 - dx)) + (q21.g * dx);
const g2 = (q12.g * (1 - dx)) + (q22.g * dx);
const b1 = (q11.b * (1 - dx)) + (q21.b * dx);
const b2 = (q12.b * (1 - dx)) + (q22.b * dx);
// Calculate the final pixel value
const r = (r1 * (1 - dy)) + (r2 * dy);
const g = (g1 * (1 - dy)) + (g2 * dy);
const b = (b1 * (1 - dy)) + (b2 * dy);
return { r: r, g: g, b: b };
}
The bilinearInterpolation
function takes the image data, the coordinates of the new pixel, and returns the interpolated pixel value. It calculates the weights based on the distance between the new pixel and the surrounding pixels, and then performs the interpolation to estimate the RGB values of the new pixel.
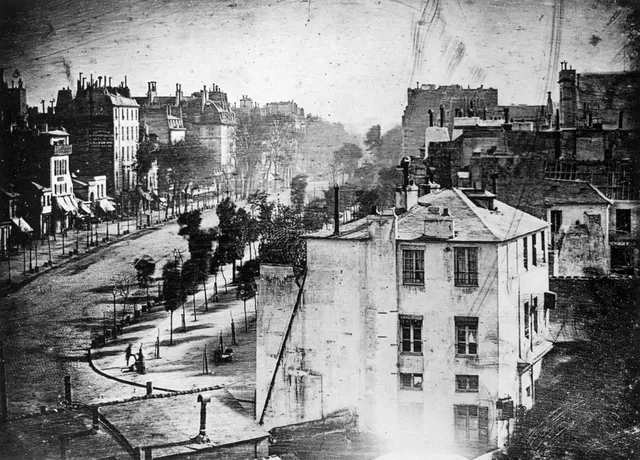
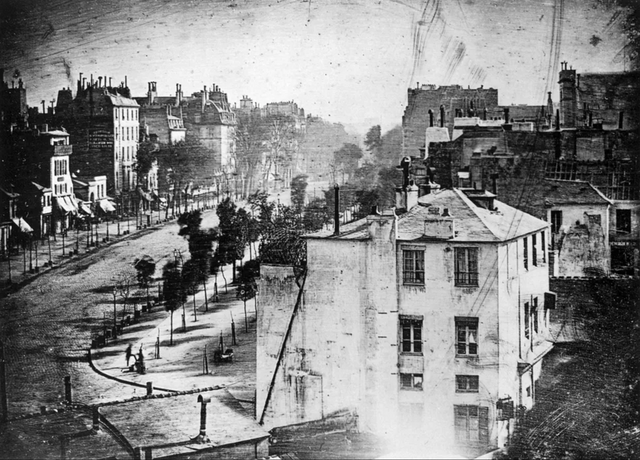
Neumorphism Effect
Neumorphism is a design trend that creates a soft, extruded appearance, resembling physical objects. To apply the neumorphism effect to the upscaled image, we can use CSS box shadows and gradients. Here's an example CSS code for the neumorphism effect:
.neumorphic {
background-color: #f0f0f0;
box-shadow: 4px 4px 8px rgba(0, 0, 0, 0.2), -4px -4px 8px rgba(255, 255, 255, 0.8);
}
In this example, the background-color
sets the base color of the element, and the box-shadow
creates the neumorphic effect by adding a darker shadow on the bottom right and a lighter shadow on the top left.
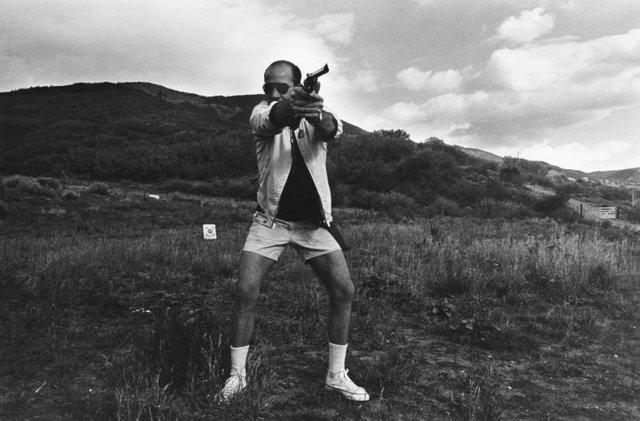
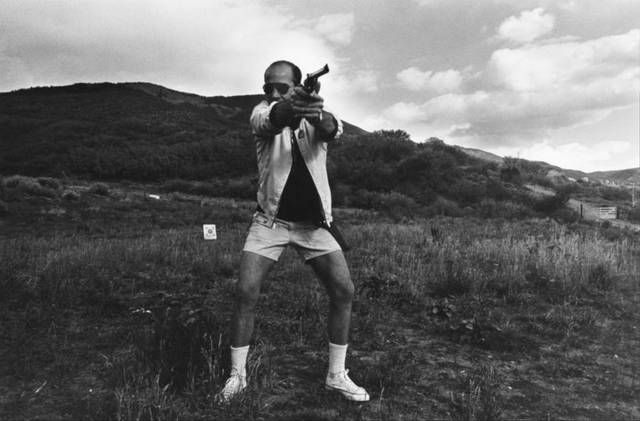
Glassmorphism Effect
Glassmorphism is a design trend that simulates the look of frosted glass or translucent materials. To apply the glassmorphism effect to the upscaled image, we can use CSS background blur, transparency, and gradients. Here's an example CSS code for the glassmorphism effect:
.glassmorphic {
background-color: rgba(255, 255, 255, 0.2);
backdrop-filter: blur(8px);
border-radius: 8px;
}
In this example, the background-color
sets the base color of the element with transparency, the backdrop-filter
applies a blur effect to create the frosted glass appearance, and the border-radius
creates rounded corners.
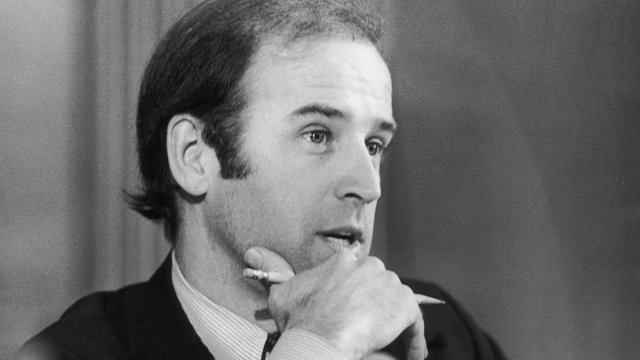
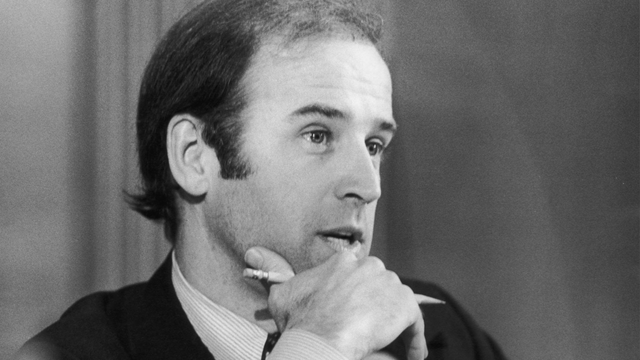
Claymorphism Effect
Claymorphism is a design trend that emulates the look and feel of clay or soft materials. To apply the claymorphism effect to the upscaled image, we can use CSS gradients and subtle shadows. Here's an example CSS code for the claymorphism effect:
.claymorphic {
background-color: #f0f0f0;
box-shadow: 2px 2px 4px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
In this example, the background-color
sets the base color of the element, the box-shadow
adds a subtle shadow, and the border-radius
creates rounded corners to enhance the clay-like appearance.
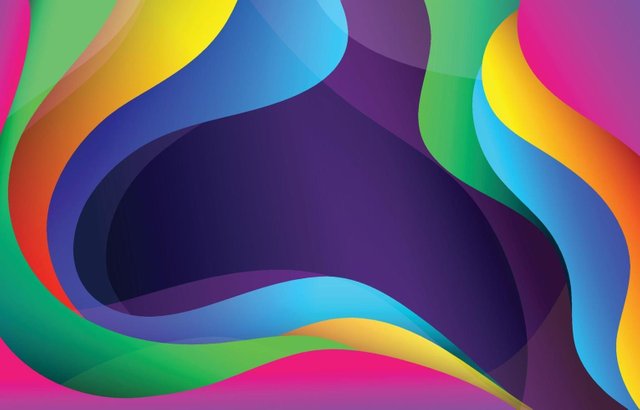
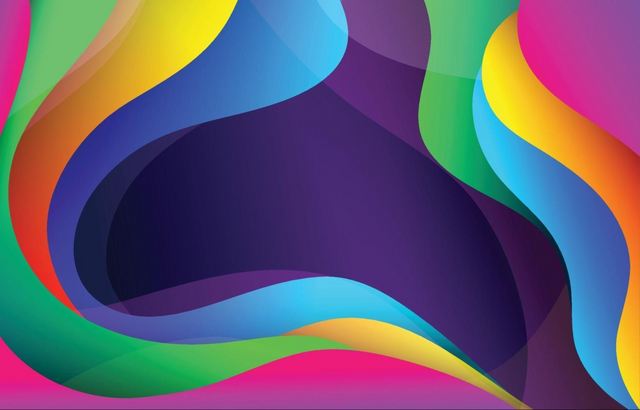
Conclusion
In this documentation essay, we explored the concept of image upscaling with bilinear interpolation and discussed how to enhance the visual appeal of the upscaled images using neumorphism, glassmorphism, and claymorphism effects. Bilinear interpolation provides a simple yet effective method for upscaling images, while the design trends of neumorphism, glassmorphism, and claymorphism add a professional and visually appealing touch to the upscaled images. By combining these techniques, you can create stunning and realistic upscaled images that captivate the viewer's attention.