Mastering File Handling Part 2: Managing Data Records with Pickle
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @kouba01 about Managing Data Records with Pickle. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
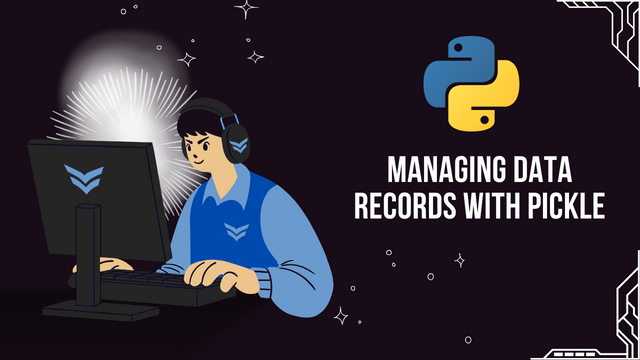.png)
Health Exercise Application
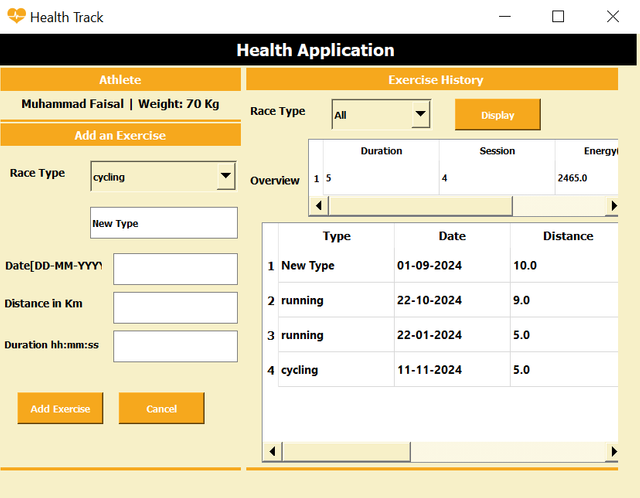
I have used these widgets for the formation of the above GUI:
Label Widgets
- First label is used to display Heart Health.
- Then similarly I have used numerous label widgets to display the instruction and hints for the user.
Input Fields
- First input field is to get the new type of the exercise.
- The next input fields are used to get input from the user for date, distance and duration.
Button
- I have used three
pushButton
. - One button is
Add Exercise
. - The other button is
Cancel
. - The last button is
Display
.
Table Widget
- I have used a
tableWidget
to display the data in the form of the rows and column.
ComboBox
- I have used two
comboBox
to select the type of the race.
Setting Custom Names
- I have set specific name for each widget used in this user interface to manage the working and functionality in the code.
This is a PyQt5-based application for managing exercise data, such as logging, storing, and viewing exercise details like type, date, distance, duration, and energy burned. Below are the major functionalities and their explanations:
Major Functions
1. populate_exercise_types()
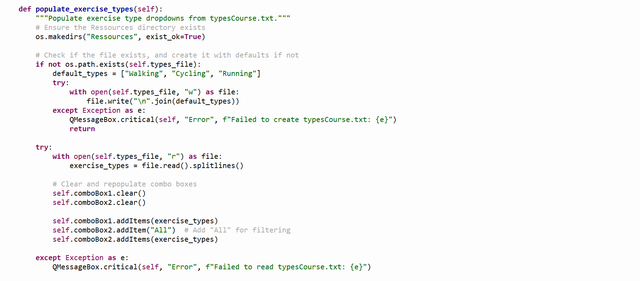
- Purpose: Initializes and updates the dropdown menus (
comboBox1
andcomboBox2
) with exercise types. - Working:
- Ensures a directory and file (
typesCourse.txt
) exist. - If the file doesn't exist, creates it with default exercise types like "Walking," "Cycling," etc.
- Reads the exercise types from the file and populates the dropdowns for adding and filtering exercises.
- Ensures a directory and file (
2. add_exercise_entry()
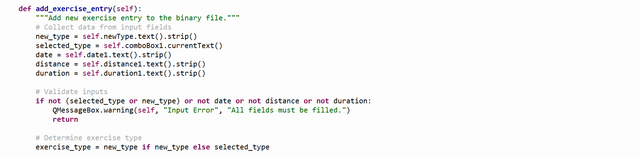
- Purpose: Collects exercise data from the input fields and stores it in a binary file (
Exercice.dat
). - Working:
- Validates user inputs for type, date, distance, and duration.
- Determines the type of exercise (new or selected).
- Converts distance and duration into energy burned using a fixed formula.
- Saves the exercise entry in the binary file:
- If the file doesn't exist, creates it.
- If it exists, appends the new data to existing entries.
- Adds new exercise types to
typesCourse.txt
if applicable. - Refreshes the dropdown menus and clears input fields after successful addition.
3. clear_input_fields()
- Purpose: Clears all the input fields in the form.
- Working: Resets text fields (
newType
,date1
,distance1
,duration1
) to empty strings.
4. display_overview()
- Purpose: Displays a summary of exercise sessions and detailed records filtered by type.
- Working:
- Reads exercise records from the binary file.
- Filters records based on the selected type (
comboBox2
), or displays all if "All" is chosen. - Calculates:
- Total duration (in seconds).
- Total energy burned.
- Number of sessions.
- Updates the overview table with these totals.
- Populates a detailed table with individual records, showing their type, date, distance, duration, and energy burned.
5. time_to_seconds(duration)

- Purpose: Converts a time string (formatted as
hh:mm:ss
) into total seconds. - Working: Splits the duration string into hours, minutes, and seconds, and computes the total seconds.
Overall Flow
Initialization:
- The UI is loaded using
loadUi()
. - Dropdown menus are populated using
populate_exercise_types()
.
- The UI is loaded using
Adding an Exercise:
- Users fill in the exercise type, date, distance, and duration.
- The app calculates energy burned and saves the data.
Viewing Exercise Data:
- Users can filter by exercise type or view all records.
- Total sessions, duration, and energy are displayed in summary.
Data Handling:
- The application uses text files (
typesCourse.txt
) for types and binary files (Exercice.dat
) for data storage. - Uses the
pickle
module for efficient serialization and deserialization of exercise data.
- The application uses text files (
This code is designed with error handling for file I/O and input validation to ensure robustness. It also provides user feedback via message boxes for success or error scenarios.
TV Channel Application
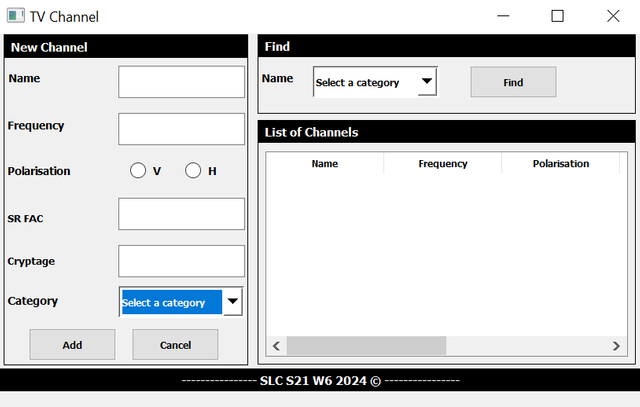
I have used these widgets for the formation of the above GUI:
Label Widgets
- First label is used to display New Channel.
- Then similarly I have used numerous label widgets to display the instruction and hints for the user.
Input Fields
- First input field is to get the name of the channel.
- The next input fields are used to get input from the user for frequency, SR FAC and cryptage.
Button
- I have used three
pushButton
. - One button is
Add
. - The other button is
Cancel
. - The last button is
Find
.
Table Widget
- I have used a
tableWidget
to display the data in the form of the rows and column.
ComboBox
- I have used two
comboBox
to select the category of the channel.
Setting Custom Names
- I have set specific name for each widget used in this user interface to manage the working and functionality in the code.
This is a PyQt5 based application for managing channel information such as frequency, polarization, and category. It uses pickle
to handle persistent storage in binary files and categories.txt
for predefined categories. Below is the explanation of major functionalities and logic:
Major Functions
1. filList
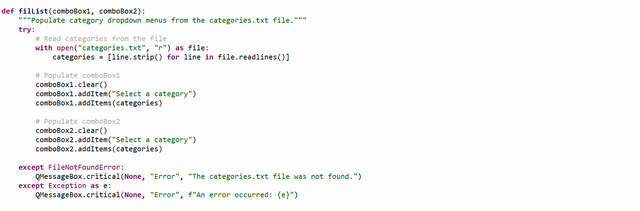
- Purpose: Populates the two dropdown menus (
comboBox1
andcomboBox2
) with categories listed in thecategories.txt
file. - Logic:
- Opens
categories.txt
and reads all lines into a list. - Clears existing dropdown options and repopulates them with the categories.
- Adds a default "Select a category" option at the beginning of both dropdowns.
- Opens
- Error Handling: Shows an error message if
categories.txt
is not found or if there is an issue reading the file.
2. validate_input
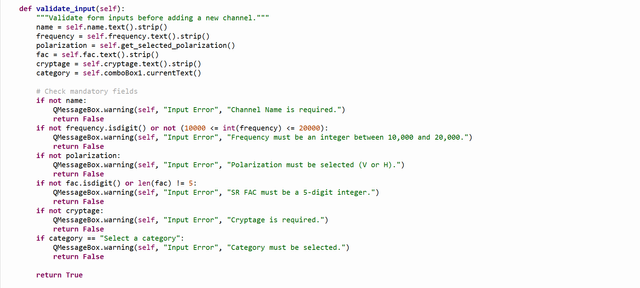
- Purpose: Ensures that all form inputs are valid before allowing the user to add a channel.
- Logic:
- Checks if mandatory fields like channel name, frequency, polarization, SR FAC, and category are properly filled.
- Ensures frequency is an integer between 10,000 and 20,000 and SR FAC is a 5-digit integer.
- Validates that a polarization option is selected (either Vertical
V
or HorizontalH
).
- Output: Returns
True
if all validations pass; otherwise, shows warning messages and returnsFalse
.
3. get_selected_polarization

- Purpose: Determines which polarization (
V
orH
) is selected from the radio buttons. - Logic:
- Returns "V" if the corresponding radio button is checked.
- Returns "H" if the other button is checked.
- Returns
None
if neither is selected.
4. add_channel
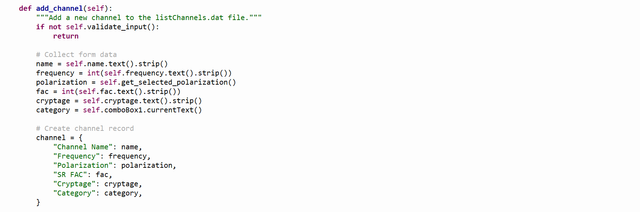
- Purpose: Adds a new channel to the
listChannels.dat
file. - Logic:
- Validates form inputs using
validate_input
. - Gathers data from the form fields and creates a dictionary to represent the channel.
- Writes the channel dictionary to
listChannels.dat
usingpickle
. If the file does not exist, it creates a new one. - Clears the form fields after successfully adding the channel.
- Validates form inputs using
- Error Handling: Displays appropriate messages if there are issues with file operations.
5. clear_form

- Purpose: Resets all input fields to their default state.
- Logic:
- Clears text inputs, resets radio buttons, and sets dropdowns to the default "Select a category" option.
6. find_channels
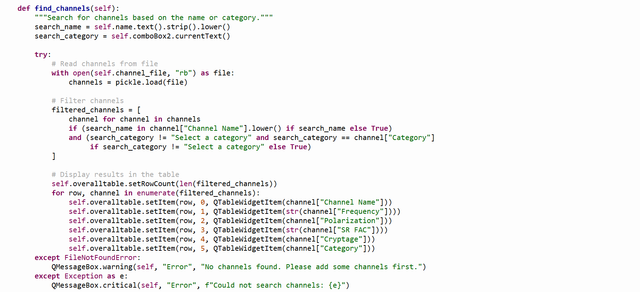
- Purpose: Filters and displays channels based on search criteria (name or category).
- Logic:
- Reads all channel records from
listChannels.dat
usingpickle
. - Filters the records:
- Matches channel names containing the search term (case-insensitive) if provided.
- Matches the selected category if it’s not "Select a category."
- Updates the table widget (
overalltable
) to display the filtered results.
- Reads all channel records from
- Error Handling: Displays messages if no channels are found or if file operations fail.
File Handling
categories.txt
:- Stores predefined channel categories.
- Populated into the dropdown menus for selection.
listChannels.dat
:- A binary file storing the list of channels as serialized dictionaries using
pickle
.
- A binary file storing the list of channels as serialized dictionaries using
Flow of the Application
- The GUI is loaded from the
channel.ui
file, and dropdowns are populated usingfilList
. - Users can:
- Add new channels via
add_channel
:- Fill in details (name, frequency, polarization, etc.).
- Validate inputs and save the channel to the file.
- Search for channels via
find_channels
:- Provide a partial name or select a category to filter records.
- View results in a table.
- Reset the form via
clear_form
.
- Add new channels via
This design makes the application intuitive for managing channel data with effective validation and file handling.
Developer Sheets Application
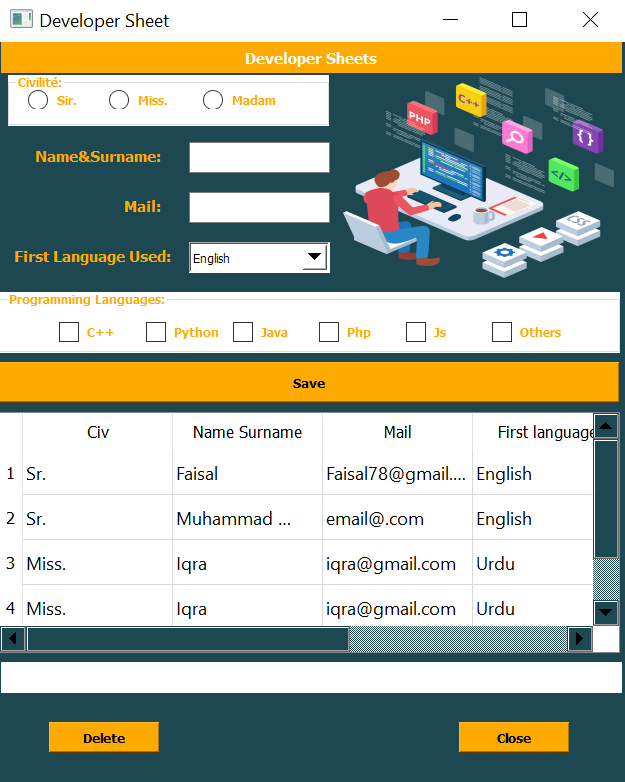
I have used these widgets for the formation of the above GUI:
Label Widgets
- First label is used to display Developer Sheets.
- Then similarly I have used numerous label widgets to display the instruction and hints for the user.
Input Fields
- I have used 2 input fields.
- First input field is to get the name and surname of the user.
- The next input field is to get the email address.
Button
- I have used three
pushButton
. - One button is
Save
. - The other button is
Delete
. - The last button is
Close
.
Table Widget
- I have used a
tableWidget
to display the data in the form of the rows and column.
ComboBox
- I have used a
comboBox
to select the first language.
Setting Custom Names
- I have set specific name for each widget used in this user interface to manage the working and functionality in the code.
The app manages records of developers, including their personal details, programming preferences, and languages known. Here's a detailed explanation:
Purpose
- Manage Developer Records: Allows users to input and save developers' details, display existing records, and reset forms.
- Persist Data: Stores the data in a binary file (
sheet.dat
) using thepickle
module. - Interactive GUI: Features user-friendly interfaces for adding and viewing records.
Major Functions
Initialization and Setup (__init__
)
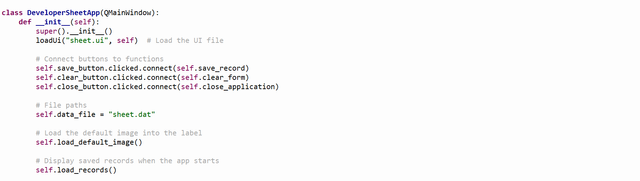
- UI Loading:
- The
sheet.ui
file defines the graphical user interface. It's loaded usingloadUi
.
- The
- Button Connections:
save_button
: Triggers thesave_record
function.clear_button
: Resets the form viaclear_form
.close_button
: Closes the application viaclose_application
.
- Default Image: Loads an image (
side_image.png
) into theimage_label
widget, scaled to fit the label size. - Load Records on Start: Reads previously saved records from
sheet.dat
and displays them in a table widget.
Image Handling

load_default_image
: Displays a default image (side_image.png
) in the GUI.- Uses
QPixmap
to load the image. - Ensures the image fits the
image_label
widget precisely usingscaled
.
- Uses
Input Validation
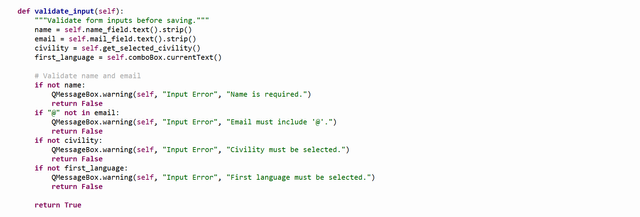
validate_input
: Ensures all required fields are correctly filled:- Name: Must not be empty.
- Email: Must contain an
@
symbol. - Civility: A radio button (e.g., "Sr.", "Miss.", "Madam") must be selected.
- First Language: A dropdown menu must have a valid selection.
- Radio Button Selection (
get_selected_civility
): Checks which civility is selected. - Programming Language Selection (
get_selected_languages
): Retrieves all programming languages marked via checkboxes.
Saving Records
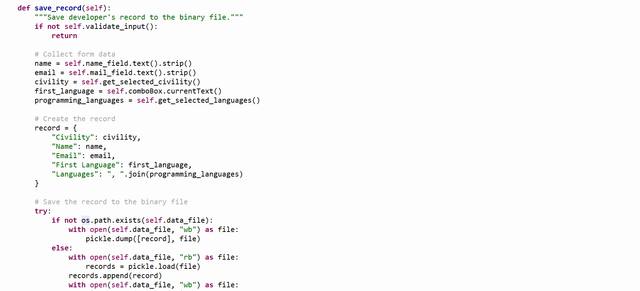
save_record
:- Data Collection: Gathers inputs from form fields.
- Validation: Ensures inputs are valid using
validate_input
. - Record Creation: Constructs a dictionary for the developer record.
- File Handling:
- If the file (
sheet.dat
) exists, it appends the new record to the existing list. - If the file doesn't exist, it creates a new file and saves the record.
- If the file (
- UI Feedback: Updates the
action_label
and displays a success message. - Error Handling: Catches and reports errors using a
QMessageBox
.
Clearing the Form
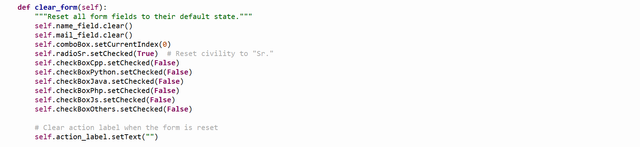
clear_form
: Resets all form fields to default values:- Text fields (
name_field
,mail_field
) are cleared. - Civility (
radioSr
) is reset to "Sr.". - Checkboxes are unchecked.
- Action label (
action_label
) is cleared.
- Text fields (
Loading Records
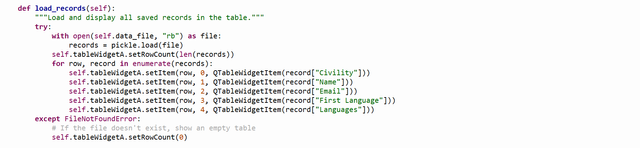
load_records
:- Reads records from
sheet.dat
usingpickle
. - Populates the
tableWidgetA
with the data:- Civility, Name, Email, First Language, and Programming Languages are displayed.
- If the file is not found, the table is shown as empty.
- Reads records from
How the Application Works
Start Up:
- The UI is loaded.
- Existing records are displayed in the table widget.
- A default image is loaded into the image label.
Adding a Record:
- Fill in the form with details like name, email, civility, first language, and programming languages.
- Click "Save" to validate inputs and save the record to
sheet.dat
.
Viewing Records:
- Saved records are displayed in the table widget.
Clearing the Form:
- Click "Clear" to reset all fields to default.
Closing the App:
- Click "Close" to exit.
This app combines input validation, file handling, and GUI features to create an efficient record management system for developers.
I would like to invite @heriadi, @chant and @jospeha to join this contest.