SLC S22 Week2 || The Object Approach In JAVA
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @kouba01 about The Object Approach In JAVA. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
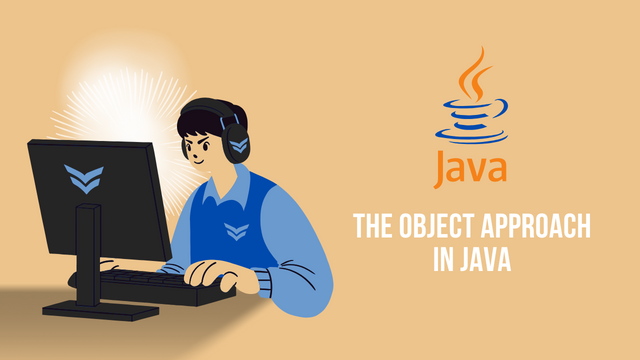
Task1
Which of the following statements about Java classes and objects is true?
Answers:
What is a class in Java?
(A) A blueprint for creating objects.
Explanation: A class in Java defines the structure and behavior that objects of that class will have. It serves as a template or blueprint for creating objects.What does the new keyword do in Java?
(B) Creates a new object of a class.
Explanation: Thenew
keyword is used to allocate memory and instantiate an object of a specified class.Which of the following statements is true about attributes in a class?
(C) Attributes store data for an object.
Explanation: Attributes, also known as fields or properties, are variables that hold data specific to an object.What is the purpose of a constructor in a class?
(B) To initialize the fields of a class.
Explanation: Constructors are special methods used to initialize the fields of an object when it is created.
Task2
Write a program that demonstrates the creation and use of a class by including attributes, methods, and object instantiation. Specifically, create a Laptop class with attributes brand (a string) and price (a double).
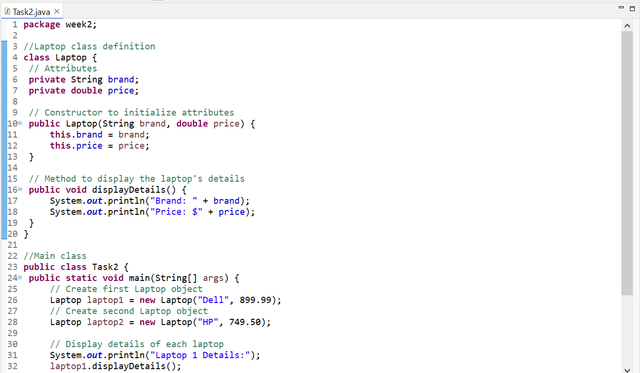
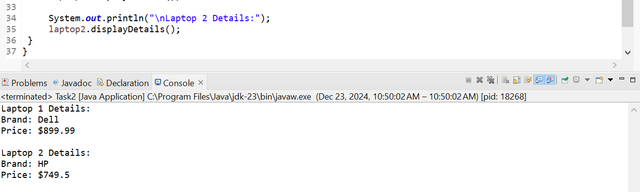
Explanation:
1. Class Definition (Laptop
)
Attributes:
I have declared two attributes of the class which are given below:
brand
: AString
that holds the brand of the laptop.price
: Adouble
that holds the price of the laptop.
These attributes are declared as private
to abide by the principle of encapsulation which prevents them from being accessed directly from outside the class.
Constructor:
- According to the requirement of the task I have then created a constructor named
Laptop
./ This will initialize the attributes at the time of creation of any object of the class. - It accepts two parameters
brand
andprice
. These are used to assign values to the attributes of the object that is being created.
Method (displayDetails
):
- This is a public method that prints the laptop details such as its brand and price.
System.out.println
is used to format and print the output.
2. Main Class (Main
)
- The
Main
class has themain
method which is the entry point of the program.
Object Creation:
There are two objects of the Laptop
class created using the new
keyword:
laptop1
is assigned with brand "Dell" and price 899.99.laptop2
is assigned with brand "HP" and price 749.50.
The constructor of theLaptop
class is called for each object and it is passing the respective values as arguments.
Calling Methods:
- The
displayDetails
method is called on each objectlaptop1
andlaptop2
to print their respective details for the user. - The output is formatted using headers such as "Laptop 1 Details:" and "Laptop 2 Details:" in order to keep the data separate for each object clearly.
3. Program Flow
- The class
Laptop
has attributes, a constructor and a method. - In the
Main
class:- Two
Laptop
objects are initiated with different values. - The
displayDetails
method is called for each object and the details are printed to the console.
- Two
- The program terminates after displaying the details of both objects.
Important Features
- Encapsulation: The
private
keyword ensures that the attributes of the class can only be accessed via methods. - Reusability: The
Laptop
class is reusable, and multiple objects with different values can be created easily. - Object-Oriented Programming: This example illustrates the basic concepts of OOP:
- Class and Object creation.
- Using a constructor for initialization.
- Encapsulation with attributes and methods.
This program is a simple but effective demonstration of how to create and use a class in Java.
Task3
Write a program that demonstrates the creation of a Movie class, its attributes, and methods, along with managing multiple objects in an array. Define the Movie class with the attributes title (a string) and rating (a double).
Here is the program that demonstrates the creation of a Movie
class, its attributes, and methods, while managing multiple objects in an array.
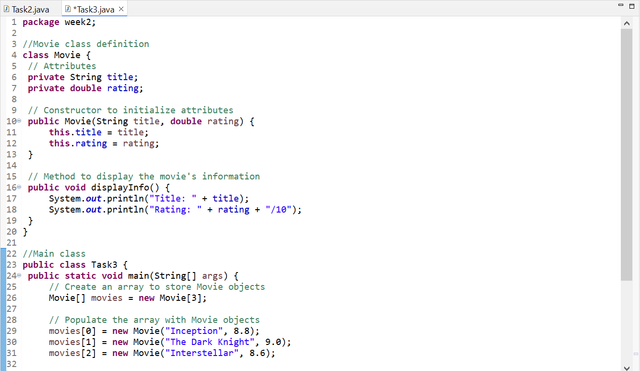
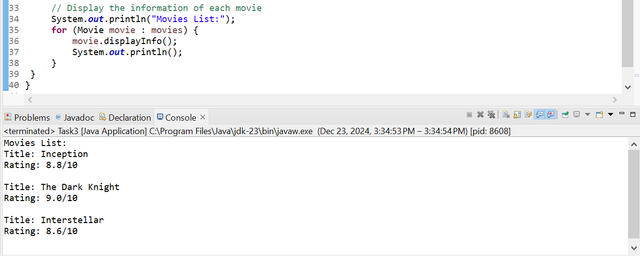
Explanation
1. Class Definition (Movie
)
Attributes:
Here in this class I have declared two attributes as follows:
title
: AString
that holds the title of the movie.rating
: Adouble
that holds the rating of the movie on a scale of 10.- Both attributes are declared private to ensure encapsulation.
Constructor:
- The
Movie
constructor takes arguments to assign values to thetitle
andrating
properties when aMovie
object is created.
Method (displayInfo
):
- The method prints out the title and rating of a movie.
- The rating is printed as a fraction of 10.
2. Main Method
Array Creation:
- A new array called
movies
of size 3 is created to hold three objects of typeMovie
.
Fill the Array:
- The array is filled with instances of the
Movie
class by using thenew
keyword. - Every object is initialized with some specific values for
title
andrating
.
**Print the Details:
- A
for-each
loop iterates through themovies
array and calls thedisplayInfo
method for eachMovie
object to print its information. - The details of each movie are printed on a new line for better readability.
Important Features
- Encapsulation: The
Movie
class employs private attributes and public methods to control and modify access. - Array of Objects: The program illustrates the management of a set of objects of a class using an array.
- Constructor Usage: An array of objects is instantiated using a parameterized constructor to make the program easy and readable.
- Iteration with Loops: The
for-each
loop simplifies accessing each object in the array.
This program explains how to manage multiple objects of a class and structure their data for efficiency and reusability.
Task4
Write a program that demonstrates adding methods with calculations by creating a Product class. The class should include the attributes name (a string), price (a double), and quantity (an integer).
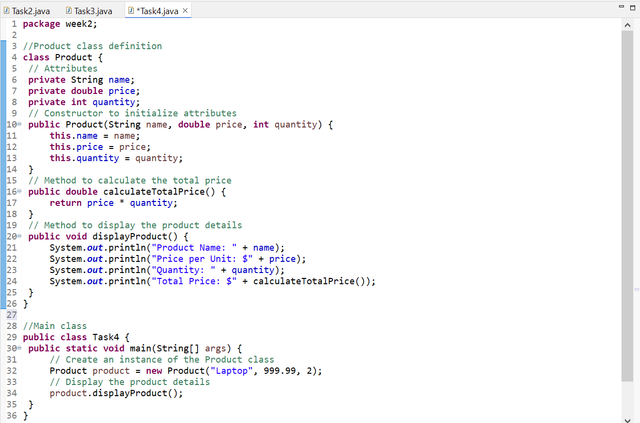

Explanation
1. Class Definition (Product
)
Attributes:
I have declared 3 attributes in the class product which are as follows:
name
: AString
that holds the name of the product.price
: Adouble
that represents the price of one unit of the product.quantity
: Anint
that holds the quantity of the product.- These attributes are private for encapsulation.
Constructor:
Then there is a constructor to initialize the attributes with values at the time of creation of a Product
object.
Method (calculateTotalPrice
):
- This is the method to calculate the total price so its presence compute the total price of the product by multiplying
price
with thequantity
of the product. - After the calculation this method return the computed value as a
double
.
Method (displayProduct
):
- Display the details of the product including its name, price per unit, quantity and total price.
- Calls the
calculateTotalPrice
method to calculate and print the total price dynamically.
2. Main Method
Object Creation:
- An object of the
Product
class is created using thenew
keyword which is then initialized with specific values forname
,price
andquantity
.
Calling Methods:
The displayProduct
method is called to print all details of the product including the calculated total price.
Key Features
- Encapsulation: The
Product
class holds all its attributes in private and has methods for accessing it. - Dynamic Calculation: The
calculateTotalPrice
method ensures the total price is calculated dynamically by current values. - Reusability: The
displayProduct
method centralizes the display logic to be reused with multiple product objects. - Real World Simulation: This program simulates the real-world concept of inventory management by merging product information with calculations.
Task5
Write a program that manages student records by creating a Student class. The class should have the attributes id (an integer), name (a string), and marks (an array of integers).
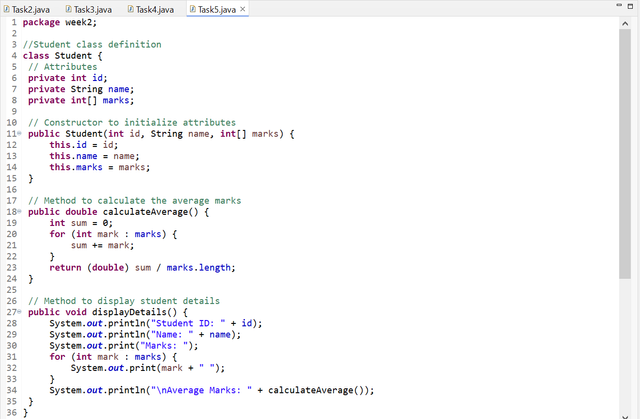
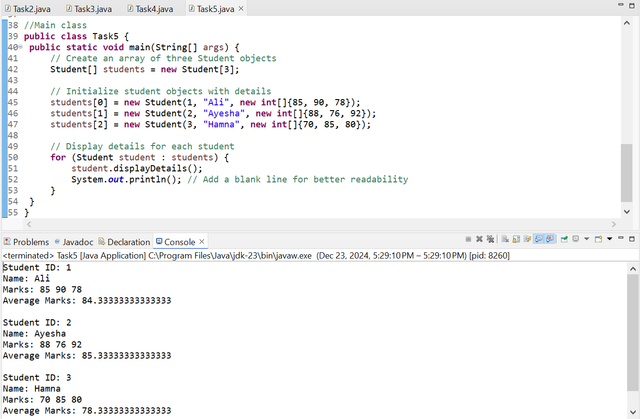
Explanation
1. Class Definition (Student
):
The Student
class encapsulates all details and functionality about a student in an attempt to embody the principles of Object Oriented Programming, namely encapsulation and modularity.
Attributes:
I have declared three attributes of the class Student which are given below:
id
: An integer uniquely identifying each student.name
: A string for the student's name.marks
: An array of integers to store the marks obtained by the student in different subjects.- These are private, thus data is accessible only through class methods.
Constructor:
- A constructor initializes the attributes when a
Student
object is created. - The
students[0]
setsid
to 1,name
to "Ali", andmarks
to{85, 90, 78}
in the first student object
Method calculateAverage()
:
The calculateAverage
method calculates the average marks of the student:
- Loop Through Marks: Iterates through the
marks
array and calculates the total sum. - Average Calculation: The sum is divided by the number of subjects, which is
marks.length
to obtain the average. - Handling Precision: It returns a
double
value in order to handle the decimal values and therefore to produce the accurate result of the averages.
Method displayDetails()
:
This method prints all the details of a student, including their:
- `id` (Student ID).
- `name` (Student's name).
- `marks` (All marks as a list).
- `average marks` (Calculated using the `calculateAverage` method).
- It uses a
for-each
loop to display all marks ensuring readability.
2. Main Method:
The main
method is the entry point of the program and demonstrates how the Student
class is used in a real-world scenario.
Array of Student
Objects:
- The program utilizes an array to hold several
Student
objects. An array makes sure all students are stored and retrieved in an efficient manner. - Every element of the array is initialized with a
Student
object. Thus unique values are given toid
,name
andmarks
.
Filling the Array:
- The array is filled with an explicit call to the
Student
constructor with appropriate parameters:
Displaying Details:
- A
for-each
loop is used to iterate through theStudent
array, calling thedisplayDetails
method for eachStudent
object. - This does guarantee the details of all the students are printed out in sequence, so that code will be scalable and easily extensible.
3. Key Features
Dynamically Computed:
- The program computes the average marks dynamically, using the
calculateAverage
method. This is an example of clean separation of concerns because each method deals with one specific task.
Encapsulation:
- The private attributes and public methods make sure that the internal data of the
Student
class is properly protected and accessed only via class methods.
Reusability:
- Methods such as
calculateAverage
anddisplayDetails
make the code modular, reusable, and easy to maintain.
Handling Multiple Objects:
- This is an example of using an array to manage multiple objects of type
Student
. It shows how OOP concepts work effectively for managing real-world entities.
Readability and Structure:
- It utilizes clean loops, correct method calls, and good output formatting to make the program legible and easy to read.
- Student Information: The first student "Ali" has her
id
,name
, andmarks
printed out - Average Marks: This program dynamically calculates and prints out Alice's average marks (
84.33
). - This cycle goes for the three students, so all records are handled without difficulties.
The program is clear, scalable and maintainable by organizing and managing the student data using OOP concepts while giving accurate results.
Task6
Write a program to simulate a simple Library Management System.
Here is the implementation of a simple Library Management System in Java:
This program implements a Library Management System with two main classes: Book
and Library
. The system makes use of a menu driven approach so that it interacts with the user and allows to manage books within the library effectively.
Class Overview
1. Book Class:
The class represents a single book in the library. This class encapsulates the attributes and methods related to the individual book operations.
Attributes:
In the Book class I have declared these four attributes:
id
(int): An ID number to identify a book. For example, when a user wants to borrow or return a book.title
(String): Title of the book.author
(String): Name of the author of the book.isAvailable
(boolean): Checks if the book is available for borrowing, or is currently borrowed. It can betrue
orfalse
.
Methods:
- Constructor:
- Initializes the book's attributes (
id
,title
,author
) and setsisAvailable
totrue
by default, indicating the book is initially available.
- Initializes the book's attributes (
borrowBook()
:- Checks if the book is available:
- If available, updates
isAvailable
tofalse
and confirms the borrow operation. - If not available, he then lets the user know that this book can't be borrowed.
returnBook()
:- Sets the variable
isAvailable
totrue
marking that the book is in the library.
- Sets the variable
displayDetails()
:- Outputs information related to the book about itself in a clean form along with its availability ("Yes" or "No").
- Accessors (e.g.,
getId()
,getTitle()
:- Provide controlled access to specific attributes.
2. Library Class:
This class is the library as a whole and encapsulates the operations to be performed.
Attributes:
This class has the following attributes:
books
(ArrayList < Book >): This is a dynamic collection ofBook
objects stored in anArrayList
. This kind of data structure allows books to be easily added, removed, and traversed.
Methods:
addBook(Book book)
:- Adds a new book to the library's collection and confirms the addition.
displayAllBooks()
:- It iterates through the
books
list and displays the details of each book using theirdisplayDetails()
method. - If the library has no books, it notifies the user.
- It iterates through the
searchByTitle(String title)
:- Searches the library for a book with a matching title (case-insensitive).
- If found displays the book's details using
displayDetails()
. - If not found informs the user.
borrowBook(int id)
:- Finds a book with the ID given.
- Calls its
borrowBook()
method if found. - If not available it prompts the user.
returnBook(int id)
:- Finds a book with the ID.
- If found calls its method
returnBook()
. - If not found then prompts the user.
3. Main Method:
This is the entry point of the program where the library system is initialized and user interactions take place.
Initialization:
- A
Library
object is created and three initial books are added using theaddBook()
method.
Menu-Driven Interface:
- A menu with five options is presented:
- View all books.
- Search for a book by title.
- Borrow a book by ID.
- Return a book by ID.
- Exit the program.
- The user selects an option, and the program performs the corresponding operation by invoking the appropriate
Library
methods.
User Interaction:
- Input is taken using a
Scanner
object. - The program deals with valid and invalid inputs gracefully by giving error messages or instructions.
Key Features and Highlights:
Object-Oriented Design:
- The program follows the principles of OOP by encapsulating data and behaviour in the
Book
andLibrary
classes. - Modular design ensures easy maintainability and extensibility.
- The program follows the principles of OOP by encapsulating data and behaviour in the
Dynamic Collection:
- The use of
ArrayList
allows the library to manage a flexible number of books without predefined size constraints.
- The use of
Interactivity:
- The menu-driven interface enhances usability by guiding the user through various library operations.
Error Handling:
- For example, borrowing or returning a non-existent book (invalid ID) triggers appropriate error messages.
Reusability:
- Methods such as
displayDetails()
,borrowBook()
, andreturnBook()
in theBook
class are reused by theLibrary
class. This encourages code reuse.
Execution Walkthrough Example:
Step 1: Initial Setup
The program begins with three books in the library:
- Book 1: 1984 by George Orwell
- Book 2: To Kill a Mockingbird by Harper Lee
- Book 3: The Great Gatsby by F. Scott Fitzgerald
Step 2: User Options
View All Books
- It shows the details of all books along with its availability status.
Search for a Book by Title
- Whenever the user searches for a title, say 1984, the program would display its details.
- If the user does a search for a nonexistent title, the program will then inform the user.
Borrow a Book by ID
- If the user inputs ID
1
, the program indicates that 1984 is borrowed. - If the book is already borrowed, it will inform the user.
- If the user inputs ID
Return a Book by ID
- If the user returns a borrowed book, for example, ID
1
, the program marks it as available. - In case the book was not borrowed or the ID does not exist, it informs the user.
- If the user returns a borrowed book, for example, ID
Exit
- Stops the program cleanly.
I would like to invite @heriadi, @chant and @jospeha to join this contest.