SLC S22 Week3 || Inheritance and Polymorphism in JAVA
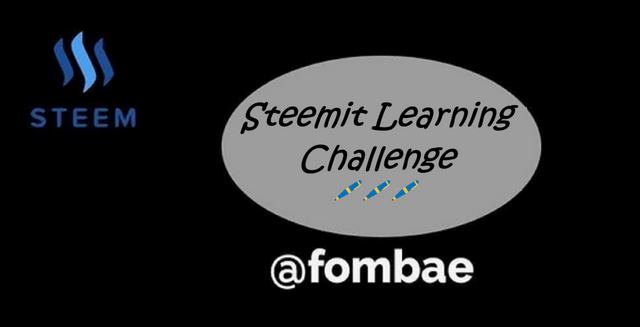
Greetings Steemit friends
Write a Java program that creates a Person class with attributes name and age. Then, create a Student class that inherits from Person and adds the attribute studentId. Demonstrate how to create objects of both classes and display their details.
Inheritance is the concept of a child class using the properties of a Parent class. This concept helps us prepare reused code and gives us the possibility to extend or override a parent class's functionality. Our task is an extendable case, where we will have to add the studentId to the parent class displaying the name and age.
Just like our previous session, we created our main class PersonDemo()
, with the class Person()
(Parent class) and the other class Student
(Child class) which will extend to the Person()
class.
The Person()
class has two attributes: name
and age
, and the constructor to initialize the attributes. Next, we have our method displayDetails()
to display the person's details.
Next, we have the Student()
class which is the child class. We have to extend to make use of the properties in the Person class. Now we can extend by adding the studentId
attribute, and override the parent class display method. The contractor is to initialize the three attributes. That is two (name and age) from the parent class and the studentId attribute.
The main class Task01()
will just be about us creating objects for Person
and Student
Classes. The Person object will carry the name
and age
, while the Student will have the additional studentId
.
Create a Shape class with overloaded methods area() to calculate the area of different shapes (circle, rectangle, and triangle) based on the provided parameters.
For us to achieve this, we will need to have different methods of Area()
(same name) to calculate the area of the different Shapes
(circles, rectangles, and triangles) in the Share()
class. So output will depend on the parameters passed.
I start by creating our main class Task2
, and next the Shape
class. In the Shape
class, we will have three different methods of the same name Area()
. One is to calculate the area of a circle (one parameter passed), the other is to calculate the area of a rectangle (two parameters passed), and the last is to calculate the area of a triangle (three parameters passed)
In the main class, an instance of the Shape
class is created. Now we can call any area method and calculate the area by passing the required parameters. Each of the methods has the formula required to calculate the area of the specific shape.
Develop a Bank class with a method getInterestRate(). Create two derived classes SavingsBank and CurrentBank that override the getInterestRate() method to return specific rates. Write a program to display the interest rates for both types of banks.
If you get my point on task one, you will have more understanding of how method overriding can be used. We were able to override the displayDetails()
for the person class with the display details for the student by extending. The same concept will work here, but this time we are returning a value. So we will have to override the value returned by the parent class Bank
since we will be asking the child class to return the new value.
I will start by creating the main class, the Bank
class (Parent), the SavingsBank
, and the CurrentBank
classes. The parent class Bank
will have the method getInterestRate()
with a default value for the interest rate. That means will have to make sure the sub-class (child classes) override and return their respective value for the interest rate.
I created SavingsBank()
and CurrentBank()
classes and extended the Bank
class. I made use of the @Override
annotation, to make sure the method is indeed an override. It is not mandatory but reduces code errors and is a good practice.
Note both classes have a method with the same name as the method in the parent class. Just like saving a new document on an old document with the same name, the new content overrides the old content, that is the concept.
In our main Class, we will create the objects for the SavingsBank
and CurrentBank
. As you can see, our output shows that the methods in the child classes override that of the Parent class and return the specific interest rates.
Create a class hierarchy with a base class Vehicle and derived classes Bike and Truck. Implement a method startEngine() in the base class and override it in the derived classes to display specific messages. Use polymorphism to call the methods through a base class reference.
From the previous task, we could see polymorphism in action. Normally overriding is a way of achieving polymorphism which is the concept used for overriding and overloading.
So just like the previous task, I will be doing the same step with change in class and method names. We are expected to use polymorphism to call the methods through a base class reference.
I created the main class, Parent class (Vehicle
), and subclasses Bike
and Truck
. Our method, in this case, is startEngine()
, which will be present in all the classes.
Here I will want to emphasize the base class reference Vehicle
which has been used to hold the Bike
and Truck
objects. In the previous task, Bank
was our base class reference to call SavingsBank
and CurrentBank
classes. Polymorphism is just the mechanism at runtime for us to achieve the overriding of a method called in the parent class.
Build a Library Management System that uses inheritance and polymorphism. Create a base class Item with attributes id, title, and isAvailable. Extend it into derived classes Book and Magazine with additional attributes. Implement polymorphic methods to borrow and return items, and display their details.
Here I will be making use of some of the code in the Library Management System from the last session. I will start by creating the main class, Item (parent) class, Book, and Magazine (child) subclasses.
In the Item
class, I declare the common attributes (id
, title
, and isAvailable
) and the method to borrow, return and display. Create the constructor, which will take three parameters id, title, and isAvailable. Next is the borrowItem()
, returnItem()
, and displayDetails()
.
Next is the subclasses Book
and Magazine
, to which I will add their specific attributes. Pretty easy because that is what we have been doing in the previous task. Each of the subclasses will have an override displayDetails()
method to display the additional attributes.
To implement Polymorphism, I used the parent class (Item
) to refer to the objects of book and Magazine. I will go ahead to call the borrowItem()
, returnItem()
, and displayDetails()
methods in the Main Class,
With the Polymorphism mechanism, it is possible to call out the borrowItem()
, and returnItem()
in the parent class using the Book and Magazine objects.
Employee Management System: An organization employs three types of workers: contractual employees, permanent employees, and hourly workers.
This task expects the system to be able to calculate worker salary and display employee details by designing a class hierarchy. With the hierarchy, I will be able to design a relationship between the different workers under the employee class. Here, we have to share the properties of the parent class with the subclasses. Hierarchy is the concept where a class (superclass) has multiple subclasses, which is the case in previous tasks above.
After creating the main Class, which will be a list of the different employee types. I design a class hierarchy starting with the base class Employee. Next is the subclass ContractualEmployee()
, PermanentEmployee()
and HourlyWorker
extend to the base class Employee
.
In the Parent Class (Employee
), the common attributes (name
, CIN
, address
, and salary
) and the placeholder method to calculateSalary()
and displayDetails()
method.
The ContractualEmployee()
subclass has the specified attribute fixedSalary
and overridden method calculateSalary()
to calculate the fixed salary.
The PermanentEmployee()
subclass has the specific attributes baseSalary
, performancesBonus
, and overridden method calculateSalary()
to calculate the total salary.
The HourlyWorker()
subclass has the specific attributes hoursWorked
, hourlyRate
, and overridden method calculateSalary()
to calculate the total salary.
Output
Task 07
This task is a multilevel inheritance, where a class inherits from another class which is a subclass to another. This is another type of class hierarchy in OOP. I will try to understand the relationship and pick out valid cases that will not have errors when compiling.
class C1 {}
class C11 extends C1 {}
class C111 extends C11 {}
- o1 = o2;
C1 o1 = new C1();
C1 o2 = new C11();
Instruction 1 is valid because o1 and o2 are references to C1 which is the parent class and no Error during runtime.
- o1 = o3;
C1 o1 = new C1();
C111 o3 = new C111();
Instruction 2 is valid, with no error because C111 is a subclass of C1
- o3 = o1;
C1 o1 = new C1();
C111 o3 = new C111();
Instruction 2 is invalid and will have an error when compiled because C1 is the parent class and not C111. The relations do not match.
- o4 = o5;
C11 o4 = new C111();
C1 o5 = new C111();
Instruction 4 is invalid and will have an error when compiled because C1 is the parent class and not C11. The relations do not match.
- o3 = (C111) o1;
Instruction 5 will be valid if the o1 refers to a C111 object.
- o4 = (C11) o5;
Instruction 6 will be valid if the o5 refers to a C11 object.
- o4 = (C111) o2;
Instruction 7 will be invalid because o2 does not refer to a C11 object but C111.
- o3 = (C11) o5;
Instruction 8 will be invalid because o5 does not refer to a C11 object but C1 making it difficult to match C111.
Task 8
To create a Geometric Inheritance system, we have to start with the Point
Class(Parent) the Rectangle
inheriting from the point class, and the Parallelogram
inheriting from the rectangle class,
Let's start with the Point
class, assign it attributes (x
and y
) and the constructor for the parameters x and y. I add the Getters and setters for x and y, and the method to toString()
Next is the Rectangle()
which inherits Points and adds specific attribute length and width. A constructor passing all the parameters. Getters and setters for length and width are included as a method to calculate the area. Finally, an override method toString()
displays details.
The last subclass is the Parallelogram()
which inherits from the rectangle class. Here we had the height attribute, a constructor and getter, and setters for height.
The area method is an override method to calculate the Parallelogram area, and next volume method, and finally the override method toString()
Output
Cheers
Thanks for dropping by
@fombae
Incredibly fast... I only managed to do half of it... I'll speed up!
0.00 SBD,
0.05 STEEM,
0.05 SP
ah, I start on Sunday. Been doing section by section as soon I have the chance. I don't think one can handle the mini projects in a day.
Upvoted! Thank you for supporting witness @jswit.