SEC S20W06 || Basic Programming Course - Lesson #6 : Functions
This is my homework post for Steemit Engagement Challenge Season 20 Week 6 assignment of Professor @alejos7ven’s class, Lesson #6 Functions.
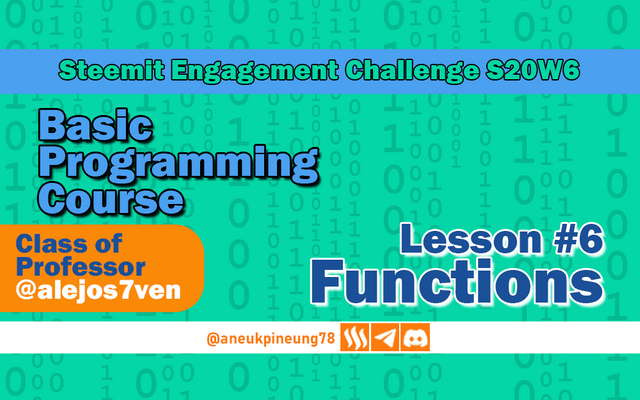
Task 1 - Tell us about your experience with programming, was it your first time? Did you find it complicated? What was your favorite part of the course?
1. My Experience With Programming
I had no previous programming experience. I liked everything related to Information Technology, especially computing, so I had subscribed to several magazines on this theme, and among those magazines there was a segment on programming and one of the magazines even gave a CD containing free programs including a programming-themed game. I have read several times to learn the structure and procedures of programming in these magazines, enough to make me say “This is not for me.”
2. Was It My First Time?
This means that so far I can understand that programming is closely related to the creation of Information Technology programs and applications, at least that's what I understand. But my involvement to learn and understand directly at a practical level only occurred in the Basic Programming class in Season 20 Steemit Engagement Challenge run by Professor @alejos7ven. This class is run very simply in a way that makes it easy for people who do not have a programming base like me to understand. Accompanied by practical tasks that are actually relevant, and perhaps because it uses pseudo-code that bridges the complexity of robotic language with simpler human language, the class becomes truly beginner-friendly.
So, yes, this is my first time in term of really learning and practicing what I learn.
3. Did You Find It Complicated?
This class is run very simply in a way that makes it easy for people who do not have a programming base like me to understand. Accompanied by practical tasks that are actually relevant, and perhaps because it uses pseudo-code that bridges the complexity of robotic language with simpler human language, the class becomes truly beginner-friendly. I did not find this class to be complicated and hard to follow at all, in fact, I felt that the class that I thought would be so complicated has been carried out in an easy and fun way.
4. My Favorite Part Of The Course
I think all parts of Professor Alejos7ven's Programming Basic class are fun. From understanding concepts, learning the structure of each concept (variables, data types, operations, control structures (conditionals and cycles and functions)), putting concepts into practice, expanding knowledge of concepts, integrating various concepts into a program, collecting additional references on the internet, it's all fun. Because in my opinion, basically it is all a unity that must be passed to truly understand and master programming. We cannot, for example, leave variables and hope that we will not face problems in understanding of the concepts.
But if I had to choose the most enjoyable part, then I would say the practicum (coding a program) as my favorite part, because coding is implementing everything we have learned into a program, it is a challenge in itself as a measure to assess how well I have understood the class.

Task 2 - Add the functions to multiply and divide to the course calculator. Explain how you did it.
1. Two new lines of code are added to the showMenu
function:
- Print “3. Multiply.”;
- Print “4. Divide.”;
2. Multiplication function is added:
Function result = multiply(n1, n2)
Define result As Real;
result = n1 * n2;
EndFunction
3. Division function is added
Function result = divide(n1, n2)
Define result As Real;
If n2 != 0 Then
result = n1 / n2;
Else
Print "Hey! Division by 0 is not possible!";
result = 0; // or other desired values;
EndIf
EndFunction
The division function checks whether the divisor (n2) is 0, because division cannot be done with a divisor of 0, if this happens then the program will display a division function failure message.
4. A case in the Switch
statement needs to be added to handle the multiplication option (command):
case 3:
n1 = askNum(n1);
n2 = askNum(n2);
result = multiply(n1, n2);
showResult(result);
reset();
5. A case to handle the division command in the Switch
statement also needs to be added:
case 4:
n1 = askNum(n1);
n2 = askNum(n2);
result = divide(n1, n2);
showResult(result);
reset();
The final result of the modified calculator program with the addition of multiplication and division functions is as follows:
Function opt = showMenu
Print "What do you want to do?";
Print "1. Sum.";
Print "2. Subtract.";
Print "3. Multiply.";
Print "4. Divide.";
Print "0. Exit.";
Read opt;
EndFunction
Function n = askNum(n)
Print "Enter a number:";
Read n;
EndFunction
Function showResult(r)
Print "The result is " r;
EndFunction
Function reset
Print "Press any key to continue.";
Wait Key;
Clear Screen;
EndFunction
Function result = sum(n1, n2)
Define result As Real;
result = n1 + n2;
EndFunction
Function result = subtract(n1, n2)
Define result As Real;
result = n1 - n2;
EndFunction
Function result = multiply(n1, n2)
Define result As Real;
result = n1 * n2;
EndFunction
Function result = divide(n1, n2)
Define result As Real;
If n2 != 0 Then
result = n1 / n2;
Else
Print "Hey! Division by 0 is not possible!";
result = 0; // or other desired values;
EndIf
EndFunction
Algorithm calc
Define n1, n2 As Real;
Define opt As Integer;
Define exit As Logic;
exit = False;
Repeat
opt = showMenu();
Switch opt Do
case 0:
Clear Screen;
Print "Bye =)";
exit = True;
case 1:
n1 = askNum(n1);
n2 = askNum(n2);
result = sum(n1, n2);
showResult(result);
reset();
case 2:
n1 = askNum(n1);
n2 = askNum(n2);
result = subtract(n1, n2);
showResult(result);
reset();
case 3:
n1 = askNum(n1);
n2 = askNum(n2);
result = multiply(n1, n2);
showResult(result);
reset();
case 4:
n1 = askNum(n1);
n2 = askNum(n2);
result = divide(n1, n2);
showResult(result);
reset();
Default:
Print "Invalid option.";
reset();
EndSwitch
While !exit
EndAlgorithm
In action example:
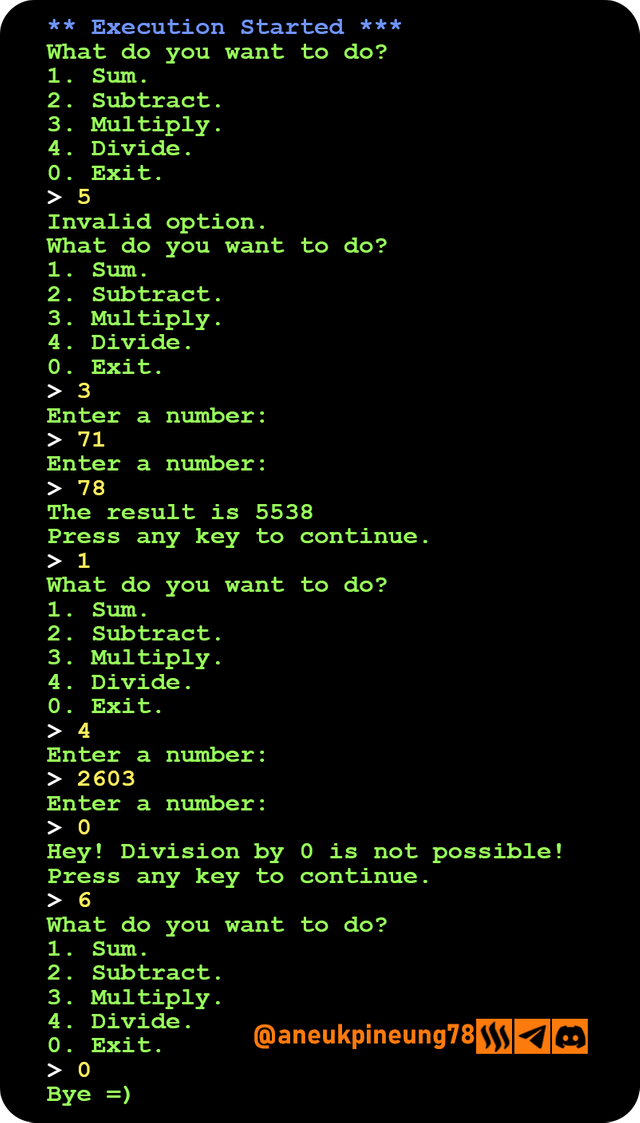

Task 3 - Create an ATM simulator. It must initially request an access pin to the user, this pin is 12345678. Once the pin is successfully validated, it must show a menu that allows: 1. view balance, 2. deposit money, 3. withdraw money, 4. exit. The cashier should not allow more balance to be withdrawn than is possessed. Use all the structures you learned, and functions.
Algorithm ATM_Sims
// 1 - Define Variables and Types of Data
Define pin, enteredPin, option, depositAmount, withdrawAmount As Integer
Define balance As Real
// 2 - Set Predefined PIN and Initial Balance
pin = 12345678
balance = 812.78
// 3 - Ask For User's PIN
Print "Enter your PIN here: "
Read enteredPin
// 4 - PIN Validation
If enteredPin = pin Then
Repeat
// 5 - Display Menu
Print "1. View Balance"
Print "2. Deposit Money"
Print "3. Withdraw Money"
Print "4. Exit"
Print "Select an option: "
Read option
// 6 - Based on the user's choice, perform relevant action
Switch Case option
Case 1:
Print "Your balance at the moment is: IDR" balance
Case 2:
Print "Enter the amount to deposit: "
Read depositAmount
balance = balance + depositAmount
Print "Deposit successful.”
Print “Your new balance is: IDR" balance
Case 3:
Print "Enter the amount you want to withdraw: "
Read withdrawAmount
If withdrawAmount <= balance Then
balance = balance - withdrawAmount
Print "Withdrawal successful.”
Print “Your new balance is: IDR" balance
Else
Print "You cannot withdraw more than what you have in balance!"
Print "Your current balance is: IDR" balance
End If
Case 4:
Print "Thank you for using the ATM. Have a nice day!"
Default:
Print "Invalid option, please try again."
End Switch
Until option = 4
Else
// 7 - Incorrect PIN
Print "Wrong PIN. Please enter the correct one."
End If
End Algorithm
The ATM simulator program has used cycle structures, conditionals and simple mathematical functions. Amongst all are:
1. Cycle structure. This structure can be seen with the use of the code Repeat ... Until
.
Repeat
// 5 - Menu Display
…
Until option = 4
This is a form of cycle where the code will continue to run until a condition is met (i.e. when the user chooses to exit by selecting option 4).
2. Conditional. The program uses If ... Then ... Else
and Switch Case
to control the program flow based on certain inputs or conditions. The program uses:
- If-Else for PIN validation:
If enteredPin = pin Then
...
Else
...
End IF
Switch Case to handle the option selected in the menu:
Switch Case option Case 1: ... Case 2: ... Case 3: ... If ... Else ... End If Case 4: ... Default: ... End Switch
If-Else to ensure the balance is sufficient for withdrawal
If withdrawAmount <= balance Then … Else … End If
3. Functions. The functions used here are simple arithmetic functions, namely addition and subtraction operations.
balance = balance + depositAmount
And
balance = balance – withdrawAmount
In action:
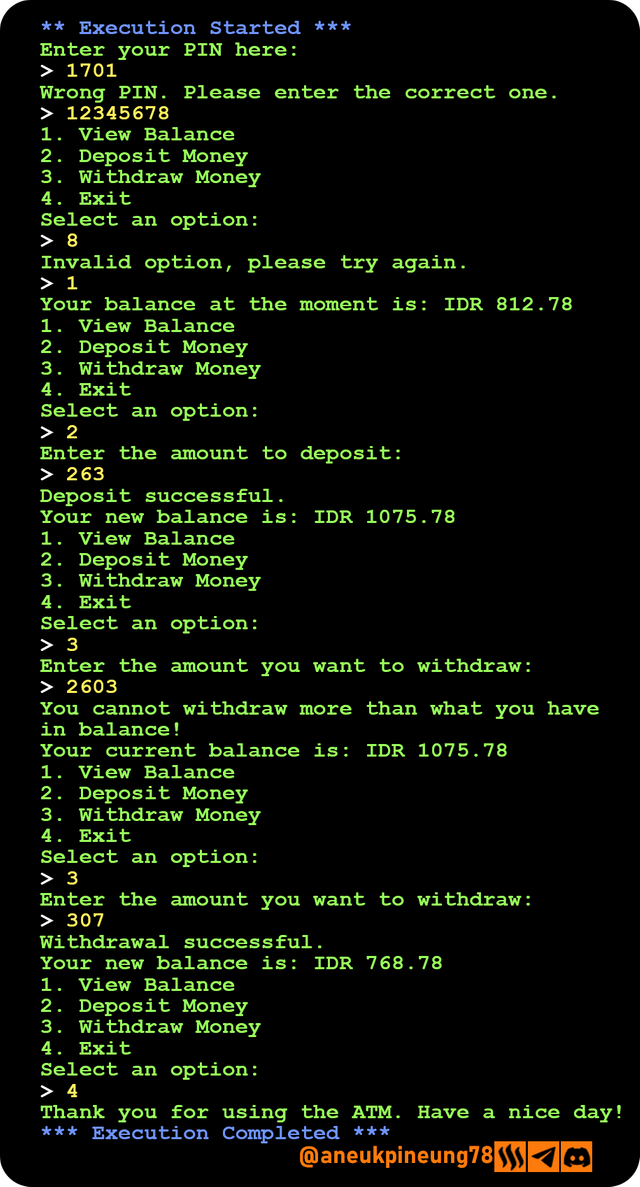

Thanks
Thanks Professor @alejos7ven for the lesson.


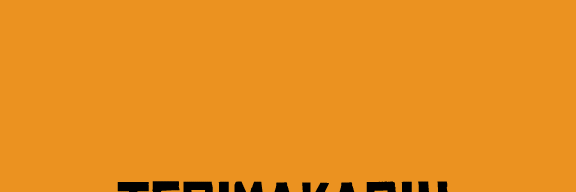

https://x.com/aneukpineung78/status/1847241246282314135
Thanks.