Tutorial - Create a dark / light mode for your website with React - Next.js
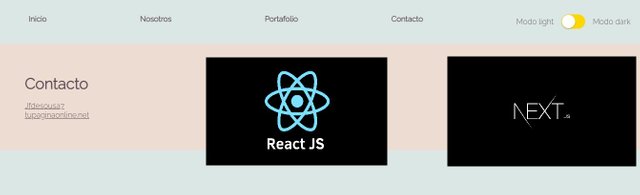
Today we are going to create a very fashionable function in these times that is to activate the light / dark mode on our website, we will use React with its Next.js Framework for this. |
We will create a folder on the desktop named toggle-dark-mode
/
Once the folder is created, we navigate to it and execute
npm init --yes
We will install the following modules:
npm i react react-dom next react-ios-switch
We will create a public /
folder and create our routes (.js)
public/index.js
import Layout from "../components/Layout";
import Head from "next / head";
const index = () => {
return (
<>
<Head>
<title>
Dark Mode | Claro in Next.js | Jfdesousa7 | yourpaginaonline.net
</title>
</Head>
<Layout>
<div className = "main-container">
<h1> Toggle Dark Mode Next.js !! </h1>
</div>
</Layout>
</>
);
};
export default index;
public/about.js
import Layout from "../components/Layout";
import Head from "next / head";
const about = () => {
return (
<>
<Head>
<title>
Dark Mode | Claro in Next.js - About | Jfdesousa7 | yourpaginaonline.net
</title>
</Head>
<Layout>
<div className = "main-container">
<h1> About </h1>
</div>
</Layout>
</>
);
};
export default about;
public/portfolio.js
import Layout from "../components/Layout";
import Head from "next / head";
const portfolio = () => {
return (
<>
<Head>
<title>
Dark Mode | Claro in Next.js - Portfolio | Jfdesousa7 | yourpaginaonline.net
</title>
</Head>
<Layout>
<div className = "main-container">
<h1> Portfolio </h1>
</div>
</Layout>
</>
);
};
export default portfolio;
public/contact.js
import Layout from "../components/Layout";
import Head from "next / head";
const contact = () => {
return (
<>
<Head>
<title>
Dark Mode | Claro in Next.js - Contact | Jfdesousa7 | tupaginaonline.net
</title>
</Head>
<Layout>
<div className = "main-container">
<h1> Contact </h1>
<br> </br>
<p> <a className="link" href="https://hive.blog/@jfdesousa7"> Jfdesousa7 </a> </p>
<p> <a className="link" href="https://tupaginaonline.net"> tupaginaonline.net </a> </p>
</div>
</Layout>
</>
);
};
export default contact;
We will create a _app.js
file which is where we will import our custom styles
public/_app.js
import '../styles.css';
// This default export is required in a new `pages / _app.js` file.
export default function MyApp ({Component, pageProps}) {
return <Component {... pageProps} />
}
We will create our .css style in the root
style.js
@ import url ('https://fonts.googleapis.com/css2?family=Raleway:wght@300&display=swap');
: root {
--font-family: 'Raleway', sans-serif;
}
*,
* :: before,
*::despues de {
margin: 0;
box-sizing: border-box;
}
.theme-dark {
--dark-text: # 292929;
--dark-background: # 2F4550;
--light-background: # 586F7C;
--accent: # b8dbd9;
--button-border: # b8dbd9;
--light-text: # F9F8F8;
}
.theme-light {
--dark-text: # 5E4B56;
--accent: # dbe7e4;
--button-border: # 5E4B56;
--dark-background: # DBE7E4;
--light-text: # 5E4B56;
--light-background: # EDDCD2;
}
body {
font-family: var (- font-family);
background-color: var (- dark-background);
color: var (- dark-text);
height: 100vh;
transition: all ease-in-out .7s;
}
nav {
background-color: var (- dark-background);
color: var (- light-text);
padding: 2em;
}
nav ul {
display: flex;
justify-content: space-between;
}
nav ul li {
list-style: none;
}
nav ul li a {
cursor: pointer;
color: var (- light-text);
font-weight: 800;
text-decoration: none;
}
.main-container {
background-color: var (- light-background);
padding: 4rem
}
.link {
color: var (- light-text);
}
@media screen and (max-width: 412px) {
nav ul {
display: flex;
flex-direction: column;
align-items: center;
gap: 1em
}
}
Components
components/Header.js
import Toggle from "../components/Toggle";
import Link from "next / link";
const Header = () => {
return (
<nav>
<ul>
<li>
<Link href = "/">
<a> Home </a>
</Link>
</li>
<li>
<Link href = "/ about">
<a> About us </a>
</Link>
</li>
<li>
<Link href = "/ portfolio">
<a> Portfolio </a>
</Link>
</li>
<li>
<Link href = "/ contact">
<a> Contact </a>
</Link>
</li>
<li>
<Toggle />
</li>
</ul>
</nav>
);
};
export default Header;
components/Layout.js
import {useEffect} from "react";
import {keepTheme} from "../utils/themes";
import Header from "./Header";
import Head from "next / head";
const Layout = ({children}) => {
useEffect (() => {
keepTheme ();
});
return (
<>
<Head>
<meta name = "viewport" content = "initial-scale = 1.0, width = device-width" />
</Head>
<Header />
{children}
</>
);
};
export default Layout;
components/Toggle.js
import {useEffect, useState} from "react";
import {setTheme} from "../utils/themes";
import Switch from "react-ios-switch";
export default function Toggle () {
const [checked, setChecked] = useState (true);
const handleOnClick = () => {
if (localStorage.getItem ("theme") === "theme-dark") {
setTheme ("theme-light");
setChecked (false);
} else {
setTheme ("theme-dark");
setChecked (true);
}
};
useEffect (() => {
if (localStorage.getItem ("theme")) {
if (localStorage.getItem ("theme") === "theme-dark") {
setChecked (true);
} else {
setChecked (false);
}
} else {
setChecked (true);
}
}, []);
return (
<div className = "light_dark">
<label>
<span className = ""> Light mode </span>
</label>
<Switch
onColor = "black"
offColor = "gold"
checked = {checked}
onChange = {handleOnClick}
/>
<label>
<span className = ""> Dark mode </span>
</label>
</div>
);
}
We will create a folder in the root called utils /
a file with two functions
utils/themes.js
export function setTheme (themeName) {
localStorage.setItem ('theme', themeName);
document.documentElement.className = themeName;
}
export function keepTheme () {
if (localStorage.getItem ('theme')) {
if (localStorage.getItem ('theme') === 'theme-dark') {
setTheme ('theme-dark');
} else if (localStorage.getItem ('theme') === 'theme-light') {
setTheme ('theme-light')
}
} else {
setTheme ('theme-dark')
}
}
And with those friends we reached the end of the tutorial, I hope you enjoyed it and until next time! |