🚀 New Protection System: Only Verified Users Can Update Malicious Voter List on Steemit 🔥Guide How to implement
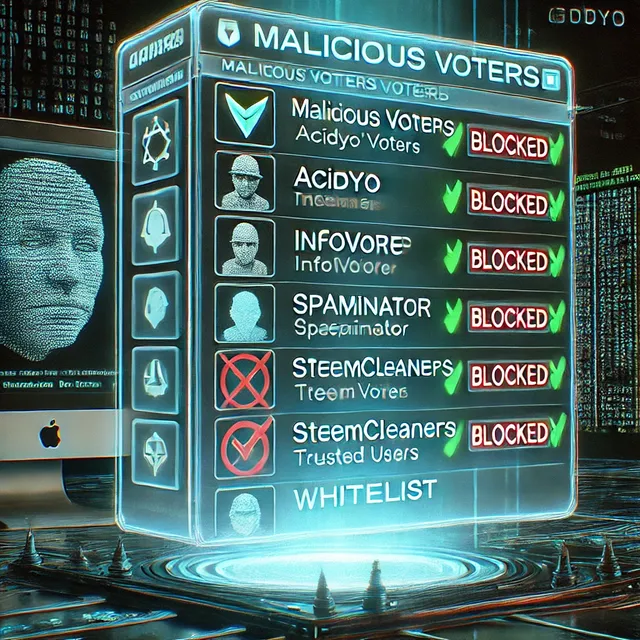
Hi all steemians and Witness and Developer.
I've been noticing for a while that this issue is being discussed a lot on Steemit and I've written a code that I want to give you that you can use to stop it.I wrote this little code for him so that he cannot cast his vote directly using the user blockchain.
I will first give you this code, then below it I will also explain in detail how you can implement it and how it can be prevented.
// ------------------- [1] Updated Hardfork Protection -------------------
#define STEEM_BLOCK_MALICIOUS_VOTES_START_TIME (fc::time_point_sec(1740682800)) // 02/27/2025 19:00 UTC
#include <unordered_set>
#include <mutex>
#include <iostream>
#include <fstream>
namespace hardforkprotect23
{
// Whitelisted users who can modify the list
inline static const std::unordered_set<std::string> trusted_users = {
"the-gorilla",
"steemchiller"
};
// Thread-safe malicious voter list
inline static std::unordered_set<std::string>& get_malicious_voters()
{
static std::unordered_set<std::string> accounts;
static std::mutex mutex_lock;
std::lock_guard<std::mutex> guard(mutex_lock); // Prevents race conditions
try
{
// Check if blockchain has a dynamic malicious list
if (database::has_dynamic_global_property("malicious_voters_list"))
{
accounts = database::get_dynamic_global_property<std::unordered_set<std::string>>("malicious_voters_list");
}
// Ensure default malicious accounts are present
accounts.insert({
"acidyo",
"infovore",
"spaminator",
"steemcleaners"
});
}
catch (const std::exception& e)
{
elog("Error fetching malicious voter list: ${e}", ("e", e.what()));
}
return accounts;
}
// ------------------- [1.1] Securely Add Malicious Voter -------------------
inline static bool add_malicious_voter(const std::string& admin, const std::string& account)
{
// Check if user is in the whitelist
if (trusted_users.find(admin) == trusted_users.end())
{
elog("Unauthorized attempt by ${admin} to add ${account} as malicious.", ("admin", admin)("account", account));
return false;
}
static std::mutex mutex_lock;
std::lock_guard<std::mutex> guard(mutex_lock);
auto& accounts = get_malicious_voters();
accounts.insert(account);
ilog("Trusted user ${admin} added malicious voter: ${account}", ("admin", admin)("account", account));
// Log the addition for security
std::ofstream log_file("malicious_voters.log", std::ios_base::app);
if (log_file.is_open())
{
log_file << "Trusted user " << admin << " added malicious voter: " << account << " at " << fc::time_point::now() << "\n";
log_file.close();
}
return true;
}
}
// ------------------- [2] Apply Operation - Vote Blocking -------------------
void database::apply_operation(const operation& op)
{
if (head_block_time() >= STEEM_BLOCK_MALICIOUS_VOTES_START_TIME &&
op.which() == operation::tag<vote_operation>::value)
{
const auto& vote_op = op.get<vote_operation>();
const auto& voter = vote_op.voter;
const auto& proxy = get_account(voter).proxy;
// Check if voter or proxy is in malicious list
if (hardforkprotect23::get_malicious_voters().count(voter) ||
hardforkprotect23::get_malicious_voters().count(proxy))
{
log_malicious_activity(voter, proxy);
FC_THROW_EXCEPTION(transaction_exception, "Vote rejected: This account is flagged as a malicious voter or proxy.");
}
}
// Continue with normal operation
operation_notification note = create_operation_notification(op);
notify_pre_apply_operation(note);
}
// ------------------- [3] Secure Logging System -------------------
void database::log_malicious_activity(const std::string& voter, const std::string& proxy)
{
static std::mutex log_mutex;
std::lock_guard<std::mutex> guard(log_mutex);
std::ofstream log_file("malicious_voters.log", std::ios_base::app);
if (log_file.is_open())
{
log_file << "Blocked vote from malicious voter: " << voter;
if (!proxy.empty()) log_file << " (Proxy: " << proxy << ")";
log_file << " at " << fc::time_point::now() << "\n";
log_file.close();
}
else
{
elog("Failed to write to malicious voters log file.");
}
}
New Usage Examples
Trusted user Add Malicious Voter
hardforkprotect23::add_malicious_voter("the-gorilla", "hacker123");
Allow Some User Update Malicious Voter List
hardforkprotect23::add_malicious_voter("the-gorilla", "hacker123");
Example of Vote Blocking
void database::apply_operation(const operation& op)
- If Malicious Voter Vote Rejected
- If Malicious Proxy Vote Rejected
Complete Guide How to update
This Updated Malicious Voting Protection System ensures that only specific and trusted users can add new names to the malicious voters list. The goal of this update is to prevent misuse or abuse and allow only verified accounts to update this list.
What is Trusted User
This update introduces a "trusted users list", which includes only those who can add new accounts to the malicious voters list.
Who Can Be in the Trusted Users List?
- Steemit core developers or moderators
- Top witnesses maintaining blockchain security
- Respected and trusted community members like @the-gorilla, @steemchiller, etc. "This is my openion"
Technical Implementation
These parameters store the lists on the blockchain, allowing dynamic updates.
#define STEEM_BLOCK_MALICIOUS_VOTES_START_TIME (fc::time_point_sec(1740682800)) // 02/27/2025 19:00 UTC
namespace hardforkprotect23
{
inline static std::set<std::string> get_trusted_users()
{
static std::set<std::string> trusted_accounts;
// Fetching from blockchain parameter storage
if (database::has_dynamic_global_property("trusted_users_list"))
{
trusted_accounts = database::get_dynamic_global_property<std::set<std::string>>("trusted_users_list");
}
else
{
// Default hardcoded list (failsafe)
trusted_accounts = {
"the-gorilla",
"steemchiller"
};
}
return trusted_accounts;
}
inline static std::set<std::string> get_malicious_voters()
{
static std::set<std::string> accounts;
// Fetching from blockchain parameter storage
if (database::has_dynamic_global_property("malicious_voters_list"))
{
accounts = database::get_dynamic_global_property<std::set<std::string>>("malicious_voters_list");
}
else
{
// Default hardcoded list (failsafe)
accounts = {
"acidyo",
"infovore",
"spaminator",
"steemcleaners"
};
}
return accounts;
}
}
Apply Operation: Vote Blocking System
Whenever a vote operation is executed, the system checks for malicious activity.
void database::apply_operation(const operation& op)
{
if (head_block_time() >= STEEM_BLOCK_MALICIOUS_VOTES_START_TIME &&
op.which() == operation::tag<vote_operation>::value)
{
const auto& vote_op = op.get<vote_operation>();
const auto& voter = vote_op.voter;
const auto& proxy = get_account(voter).proxy;
// Malicious voter check (direct + proxy)
if (hardforkprotect23::get_malicious_voters().count(voter) ||
hardforkprotect23::get_malicious_voters().count(proxy))
{
FC_THROW_EXCEPTION(transaction_exception, "Vote rejected: This account is flagged as a malicious voter or proxy.");
}
// Future scope: Add vote pattern analysis here
}
// Proceed with normal operation
operation_notification note = create_operation_notification(op);
notify_pre_apply_operation(note);
}
Allow Only Trusted Users to Update Malicious List "Already Explain"
Only trusted users (like @the-gorilla and @steemchiller) can add or remove accounts from the malicious voters list.
void database::update_malicious_voter_list(const std::string& updater, const std::string& voter, bool add)
{
// Check if updater is in trusted users list
if (!hardforkprotect23::get_trusted_users().count(updater))
{
FC_THROW_EXCEPTION(transaction_exception, "Update failed: Only trusted users can modify the malicious voter list.");
}
auto& malicious_list = hardforkprotect23::get_malicious_voters();
if (add)
{
malicious_list.insert(voter);
}
else
{
malicious_list.erase(voter);
}
// Store the updated list on the blockchain
database::set_dynamic_global_property("malicious_voters_list", malicious_list);
}
All blocked votes are logged for auditing and security purposes.
void database::log_malicious_activity(const std::string& voter)
{
std::ofstream log_file("malicious_voters.log", std::ios_base::app);
log_file << "Blocked vote from malicious voter: " << voter << " at " << fc::time_point::now() << "\n";
log_file.close();
}
I think I've written almost all the code here and have also almost given a guide on how this could be implemented.
If you have any question
Menstion Some Users
I think I've written almost all the code here and have also almost given a guide on how this could be implemented.
If you have any question
Menstion Some Users
If you have any question
Sorry, but I'm convinced that you didn't develop the code you wrote here and in your other post, and probably don't understand it either.
Some essential methods are missing and you are calling some that don't exist. Have you compiled and tested the code?
This user is our "friend" Aftab...
Mehrfach (mit diversen Accounts) aufgefallen und er kann's einfach nicht lassen.
Und weiter geht's mit der Bettelei...
What is your problem with me?
...and he asks rme for a delegation, very mysterious - that rings certain bells for me.
I shared the post in the witness channel and rme replied there and here. I guess he thought the post was from me, because he asked me the same. I explained it in the channel and I think there will be no delegation.
I didn't ask for delegation to report myself, but rather to upvote accounts that are downvoted. The rest is up to you.
I appreciate your feedback. The code was developed by me, and I have tested it before posting. If you found any missing methods or errors, feel free to point them out. I'm always open to improving and discussing the logic behind it.
Funny guy!
I don't have time to respond to your comments, so you should do what you're doing.
Let me put it this way: Your intention was good, but the result was unfortunately useless.
https://github.com/steemit/steem/compare/main...SteemLabs:steem:0.23.1-test
What do you want to say with this link?
I think you can understand yourself.
goody By
Niece to meet you I am busy at this time
good initiative. I think @abuse-watcher / @bangla.witness must be in the "Whitelisted users who can modify the list". Because, Steemit Abuse Watcher Team plays a vital role to cleanup abuses in Steemit Platform.
@rme
I'm so glad you read my post, maybe you're right, you've been working on steemit for a long time.
It was the best solution we could stop it but in my opinion and according to my knowledge we can't because Steemit is a blockchain base app if we make such changes the value of steemit will go down due to decentralization people will lose trust in it.
First of all we have to contribute to these users i.e. make some kind of deal with them so that they stop doing this.
On this I would like to say a sentence that to save all the rolls we have to break some rolls.
If we make such strictures, many users will withdraw their funds from steemit and this steemit will drop.
If we look at the current situation, the stem is far below the hive token.
Some information
If the Steam Team delegate sp any account To upvote an account that has been downvoted.
So the Steemit team will generate new tokens to delegate to the account which will further depreciate the price of Steemit.
What should we do in this situation and what steps should we take?
A top account like yours has to give me a delegation so that I can upvote accounts that are downvoted.
Delegate @security.block
I think Steemit Proposal is best rather than development funding through steem delegation. Please, create a proposal & try to pass it.