SLC S22 Week1 || Getting Started with Java and Eclipse
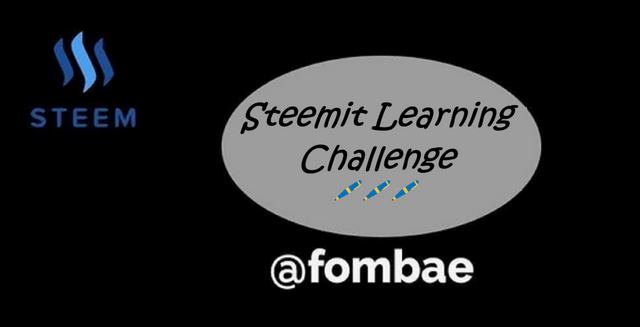
Greetings Steemit friends
Write a detailed explanation of the differences between JDK, JRE, and JVM.
JDK (Java Development Kit), JRE (Java Runtime Environment), and JVM (Java Virtual Machine) are the three main components of Java. Each component has a specific function and is combined to develop and execute an application.
- JDK (Java Development Kit)
***Roles: *** JDK provides the environment for developers to write, compile, and debug Java applications with all the necessary toolkits. JDK is made up of Compiler, debugger, and JRE (Java Runtime Environment). The compiler helps to convert source code, the debugger helps find errors in code and JRE runs the compiled program.
Functionalities: JDK is the environment for developers to write Java code, test, and compile the application.
Interdependence: JDk is a supper set for all the other components in Java comprising all the packages for building and running Java applications.
- JRE (Java Runtime Environment)
Roles: JRE, from the full mean provides the runtime environment for developers to execute Java applications. JRE is the main source of Java libraries needed to enable runtime. The runtime libraries support I/O files, and JVM to run bytecode for a Java application.
Functionalities: JRE is the environment needed to run applications without the need for a development tool.
Interdependence: JRE is a subset/element of the JDK. Developers need the JRE within the JDK to test their applications during development.
- JVM (Java Virtual Machine)
Roles: JVM's role is to provide developers with the underlying hardware and operating system to run Java applications. JVM is responsible for loading the .class files and making sure bytecode is up to date with Java security and rules.
Functionalities: JVM helps to convert bytecode into machine code and execute using interpretation.
Interdependence: JVM is a subset of JRE. The JVM is carried out in functions within the JRE, and it is the driving force to run Java bytecodes.
Install and Set Up Your Java Development Environment and provide step-by-step screenshots of the installation process, Eclipse configuration.
Let's start by visiting Oracle's official website, note it is important to always download your application from the official website. Download the JDK from official website
Verify my installation
It is imported to download from the Eclipse official website. You find the installer, Note this is not the Eclipse IDE we are expected to download. I will try to be very detailed about what I have done.
I have selected to download the installer.
Now click download.
Your download file should be in your download directory. Run the Eclipse installer. You have to scroll down to find Eclipse IDE for Java and DSL Developers. This is what is expected of this course because you will find other Eclipse packages.
Select Eclipse IDE for Java and DSL Developers. by clicking on it, and the next pop-up will give the option to install.
Click install and wait for a while. You will need an internet connection for a complete download of the Eclipse IDE for Java and DSL Developers to your laptop.
When the installation is complete, the color changes from orange to green and you can not click lunch to run the application.
One last thing I noticed was the popup of a window by Microsoft Windows Defender. The option you will select will depend on you and your preferences for running the .exe application on your devices. If you are a beginner, I would prefer you select the second option, in order to stay at some level of security on your computer.
Steps to Create a Project
File -> New -> Java project
Name the project, click next, and click finish on the next pop-up.
Step to Create a Class
File -> New -> Class.
Here, we give our program a name and have the option to select the method you want to be auto-created for you. You can click finish.
To run our program, we click the green icon directly or follow the highlighter sections on the image below.
Test out the first program by printout "Hello Steemians"
Write a program that calculates and displays the sum of the first 100 integers. Define the main method in a class named Sum to handle the entire calculation.
To create our program, we click the file, new, and select the class to create the Sun.java. The file auto-creates the public class with the name Sum.
To get the sum, we start by declaring the variable to hold the final total sum. I used the loop to iterate within the range of 1 to 100, adding each number to get the final total sum.
Now the second case is about us factorizing the code. Here I have created the function calculateSum() to have the program operations that can be reused. A parameter is specific to which number of sums we want to calculate.
In the main method, we can declare a variable, which I can sum and call the function calculateSum(). Now I have passed 100 as an argument, which will be calculated and summed return, and stored in the variable.
Translate, Complete, and Debug the Following Program Named Tax.java
Here, we have to use the if-else conditions to set the different logic for each income bracket. Each income bracket had a specific rate assigned to it. To be able to have the total tax of 5550, we need to add the result of T1 and T2.
T1: 20000 * 0.05 = 1000
T2: (40000 – 20000) * 0.10 = 2000
T3: (57000 – 40000) * 0.15 = 2550
Total Tax: 1000 + 2000 + 2550 = 5550
Using the same program structure as in the previous exercise, write a program named Conversion.java that takes a character c as a parameter
Using a similar structure as in the previous exercises, write a program named ArrayOperations.java that performs operations on an integer array.
Here I will start with the functions(methods) to handle the operations. I create the findLargest() to iterate through the array, get the largest elements, and print out the result. Next is the calculateSum() to calculate the sum by iterating through all the elements in the array and printing the result. Finally, the sortArray() to sort the array in ascending order.
Let's test our program
Now let's test the case of an empty array, and see if the handling of empty input working correctly.
Cheers
Thanks for dropping by
@fombae
Upvoted! Thank you for supporting witness @jswit.
@tipu curate
Upvoted 👌 (Mana: 2/7) Get profit votes with @tipU :)
Wow, I often see you always follow the programming language lessons, so of course you are proficient in various programming languages, one of which is the java program, I have read and seen that in your post you really have explained the material well and clearly.
You have provided clear steps in installing the Java program can run well to complete the 6 tasks given by Prof. Kouba01. Good luck my friend.