[#3] How to read Sensors | Philips Hue - C# Programming
How to read Sensors
Previous Post
In the last tutorial, I showed how to control the lights.
You can find it here: [#2] How to control Hue Lights | Philips Hue - C# Programming
Sensors
You will find a lot more than lights in the Philips Hue System, called sensors. This tutorial is limited to the Hue Motion Sensor and Hue Dimmer Switch because I don't own a Hue Tap Switch. I will focus on the motion sensor which has a built-in temperature sensor. I will show how to get the sensor data, parse it into an object and show the temperature.
Lets create the sensor template:
private const string SensorUrlTemplate = "http://{0}/api/{1}/{2}";
Now use the GetRequestToBridge from the last Tutorial:
string result = GetRequestToBridge(string.Format(SensorUrlTemplate, BridgeIP, Usercode, "sensors"));
As result, you will get all sensors from your hue system formatted as JSON. Every sensor has different properties so it's a little bit hard to parse this JSON. I created a helper class for parsing (http://json2csharp.com/) and modified it:
using System;
using System.Collections.Generic;
using Newtonsoft.Json;
using HueTestForms.Enumerations;
namespace HueTestForms.Helpers
{
public partial class SensorHelper
{
public string ID { get; internal set; }
public SensorTypes SensorType { get; set; }
[JsonProperty("state")]
public SensorHelperHelperState State { get; set; }
[JsonProperty("config")]
public SensorHelperHelperConfig Config { get; set; }
[JsonProperty("name")]
public string Name { get; set; }
[JsonProperty("type")]
public SensorTypes Type { get; set; }
[JsonProperty("modelid")]
public string Modelid { get; set; }
[JsonProperty("manufacturername")]
public string Manufacturername { get; set; }
[JsonProperty("swversion")]
public string Swversion { get; set; }
[JsonProperty("uniqueid")]
public string Uniqueid { get; set; }
[JsonProperty("recycle")]
public bool Recycle { get; set; }
[JsonProperty("swupdate")]
public SensorHelperHelperSwupdate Swupdate { get; set; }
}
public partial class SensorHelperHelperConfig
{
[JsonProperty("on")]
public bool On { get; set; }
[JsonProperty("configured")]
public bool Configured { get; set; }
[JsonProperty("sunriseoffset")]
public long Sunriseoffset { get; set; }
[JsonProperty("sunsetoffset")]
public long Sunsetoffset { get; set; }
[JsonProperty("reachable")]
public bool Reachable { get; set; }
[JsonProperty("battery")]
public long Battery { get; set; }
[JsonProperty("pending")]
public List<object> Pending { get; set; }
[JsonProperty("flag")]
public bool Flag { get; set; }
[JsonProperty("alert")]
public string Alert { get; set; }
[JsonProperty("ledindication")]
public bool Ledindication { get; set; }
[JsonProperty("usertest")]
public bool Usertest { get; set; }
[JsonProperty("sensitivity")]
public long? Sensitivity { get; set; }
[JsonProperty("sensitivitymax")]
public long? Sensitivitymax { get; set; }
[JsonProperty("tholddark")]
public long? Tholddark { get; set; }
[JsonProperty("tholdoffset")]
public long? Tholdoffset { get; set; }
}
public partial class SensorHelperHelperState
{
[JsonProperty("daylight")]
public object Daylight { get; set; }
[JsonProperty("lastupdated")]
public string Lastupdated { get; set; }
[JsonProperty("status")]
public long Status { get; set; }
[JsonProperty("buttonevent")]
public long Buttonevent { get; set; }
[JsonProperty("temperature")]
public double Temperature { get; set; }
[JsonProperty("presence")]
public bool Presence { get; set; }
[JsonProperty("lightlevel")]
public long Lightlevel { get; set; }
[JsonProperty("dark")]
public bool Dark { get; set; }
}
public partial class SensorHelperHelperSwupdate
{
[JsonProperty("state")]
public string State { get; set; }
[JsonProperty("lastinstall")]
public object Lastinstall { get; set; }
}
public static class SensorHelperHelperSerialize
{
public static string ToJson(this SensorHelper self) => JsonConvert.SerializeObject(self, SensorHelperHelperConverter.Settings);
}
public class SensorHelperHelperConverter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
};
}
}
As I mentioned in the beginning, I don't own a Hue tap switch so maybe the parsing will fail if you own one.
For the Type I added an enum:
public enum SensorTypes
{
Daylight,
CLIPGenericFlag,
ZLLSwitch,
CLIPGenericStatus,
ZLLTemperature,
ZLLPresence,
ZLLLightLevel,
}
For parsing:
public partial class SensorHelper
{
public static List<SensorHelper> FromJson(string json)
{
var dict = JsonConvert.DeserializeObject<Dictionary<string, SensorHelper>>(json, SensorHelperHelperConverter.Settings);
var result = new List<SensorHelper>();
foreach (var item in dict)
{
item.Value.ID = item.Key;
result.Add(item.Value);
}
return result;
}
}
I deserialize it to a dictonary>string, Sensorhelper> to keep the ID as Key. Afterwards I create a List of SensorHelper and add the ID to every object. I do this to have the ID in the object and don't have to handle with a dictonary.
Now it's easy to get the Temperature:
SensorList.Where(s => s.State.Temperature != 0).First().State.Temperature;
The temperature is stored as int so you have to add the comma!
If you have any questions do not hesitate to contact me per comment 😃
Geggi632
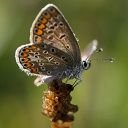