SEC-S20W6 Practice cycles and Guess the number game
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
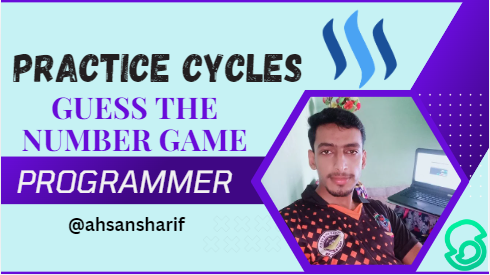
Canva Work
Escape Codes
In programming, we use ESC to set the colors of our text and to change its background. First of all, let us know what ANSI escape codes are. These are codes that are interpreted by terminal emulators to control format colors or various output options. All these codes are widely used in command-line interfaces in programming.
Structure of ESC:
\e
or \033
: These indicate our escape character. Followed by:
[
This is to start by controlling our sequence. And then:
;
: We use it to separate a sequence of numbers. Finally:
m
: Finally we use it to override our color or style control sequence.
For example
\e[31m: Sets the text color to red.
\e[0m: Resets all formatting back to default.
Here are some basic escape color codes.
Foreground / Standard Text Color
Code | Color |
---|---|
\e[30m | Black |
\e[31m | Red |
\e[32m | Green |
\e[33m | Yellow |
\e[34m | Blue |
\e[35m | Magenta (purple) |
\e[36m | Cyan (light blue) |
\e[37m | White |
BG Colors
Code | Color |
---|---|
\e[40m | Black background |
\e[41m | Red background |
\e[42m | Green background |
\e[43m | Yellow background |
\e[44m | Blue background |
\e[45m | Magenta background |
\e[46m | Cyan background |
\e[47m | White background |
Bright Bold Version Colors
Code | Color |
---|---|
\e[90m | Bright Black (Gray) |
\e[91m | Bright Red |
\e[92m | Bright Green |
\e[93m | Bright Yellow |
\e[94m | Bright Blue |
\e[95m | Bright Magenta |
\e[96m | Bright Cyan |
\e[97m | Bright White |
In addition to colors, ANSI escape codes allow you to modify text styles
Text Style
Code | Color |
---|---|
\e[1m | Bold |
\e[4m | Underline |
\e[5m | Blink (on some terminals) |
\e[7m | Inverted colors (swap foreground and background) |
\e[0m | Reset all styles and colors to default |
You can combine multiple ANSI codes in a single escape sequence by separating them with semicolons (;)
Combine Code
Code | Color |
---|---|
\e[1;31m | Bold red text |
\e[4;32m | Underlined green text |
\e[7;34m | Inverted blue text (swaps foreground and background) |
Here is an example of a C++ program where I put different colors and styles.
#include <iostream>
using namespace std;
#define RESET "\e[0m" // Reset all attributes
#define BOLD "\e[1m" // Bold
#define UNDERLINE "\e[4m" // Underline
// Foreground colors
#define BLACK "\e[30m" // Black
#define RED "\e[31m" // Red
#define GREEN "\e[32m" // Green
#define YELLOW "\e[33m" // Yellow
#define BLUE "\e[34m" // Blue
#define MAGENTA "\e[35m" // Magenta
#define CYAN "\e[36m" // Cyan
#define WHITE "\e[37m" // White
// Background colors
#define BGBLACK "\e[40m" // Black background
#define BGRED "\e[41m" // Red background
#define BGGREEN "\e[42m" // Green background
#define BGYELLOW "\e[43m" // Yellow background
#define BGBLUE "\e[44m" // Blue background
#define BGMAGENTA "\e[45m" // Magenta background
#define BGCYAN "\e[46m" // Cyan background
#define BGWHITE "\e[47m" // White background
int main()
{
cout << BOLD << RED << "This is bold red text!" << RESET << endl;
cout << UNDERLINE << GREEN << "This is underlined green text!" << RESET << endl;
cout << BLUE << BGBLUE << "This is blue text with a blue background!" << RESET << endl;
cout << YELLOW << BGMAGENTA << "This is yellow text with a magenta background!" << RESET << endl;
cout << BOLD << CYAN << BGWHITE << "This is bold cyan text with a white background!" << RESET << endl;
return 0;
}
Here is the output of this code.
Bold Red text.
Underlined Green text.
Blue text on a Blue background.
Yellow text on a Magenta background.
Bold Cyan text on a White background.
Here is the C++ Code For Guess The Number.
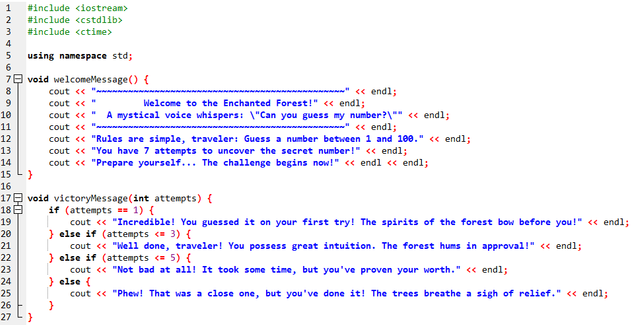
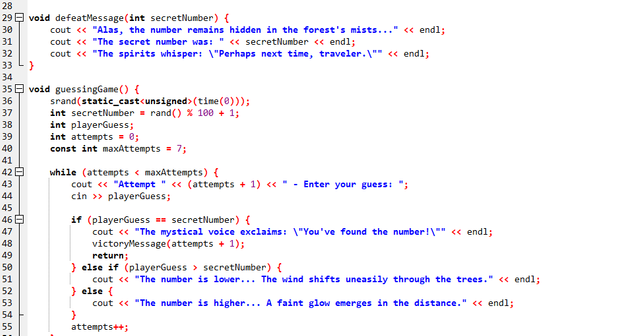
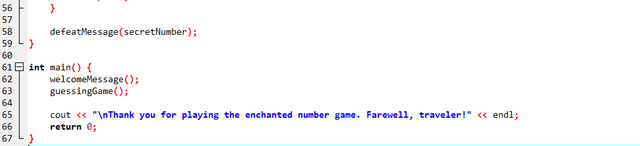
Explanation:
This is a game that is set in an enchanted forest that gives a mystical sound to the player to choose any number. And challenges him to choose the correct number.
In this game, the player will have seven chances to choose one of the correct numbers.
This is a game that offers feedback with every guess.
As soon as the player selects any number, a message will be shown on his victory. Even if he fails, he will get a message to encourage him.
It is a game that gives us a different kind of experience and completes this adventure using language.
Here is the output:
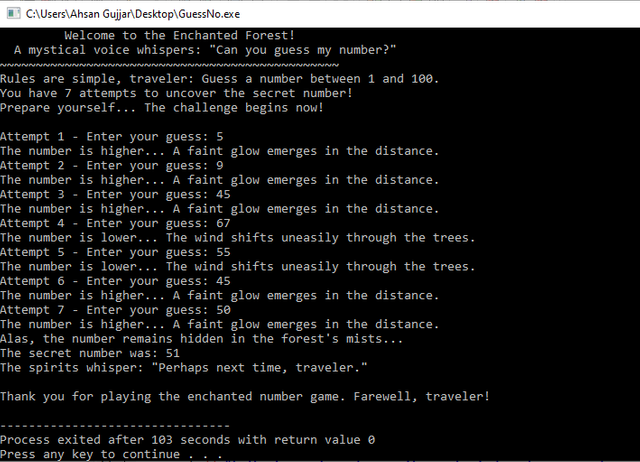
If we try to guess the number from zero to thousand or from zero to 10 thousand, it will take us a lot of time. We have two types of strategies for how to solve this.
Our first strategy will be one in which we have to make a random guess. Random guess means that we have to search for random numbers from zero to 1000 and from zero to 10,000.
This procedure is very time taking procedure. According to an average which is from zero to a thousand we have to make about 500 guesses to guess our number then we will be able to guess our correct number.
Similarly, which is from zero to 10 thousand, according to an average, we have to guess about five thousand. Then we will be able to find our correct number.
The second method we have is that we will find it according to the binary search which we can also call the optimal strategy.
If we were to use a binary search strategy where you systematically cut the range of possibilities in half with each guess. This has the advantage of reducing the number of guesses required.
Here we can calculate the number of estimates using the log formula. A log formula that has two logarithmic ranges.
Here is the log formula that we use.
0 to 1000: log2(1000)
Similarly, if we take out our range from zero to thousand, then approximately our guesses are 10.
0 to 10000: log2(10,000)
Same, if we use the log formula, our range from zero to 10,000 will have approximately 14 guesses.
Finally, we agree that if we do a random guess it will take us a lot of time and it will be a very long procedure. But if we use binary search, we will find our number very quickly. From zero to thousand there are only 10 guesses and from zero to 10 thousand there are only 14 guesses. In this range, we can find our number.
Here is the C++ Program Code
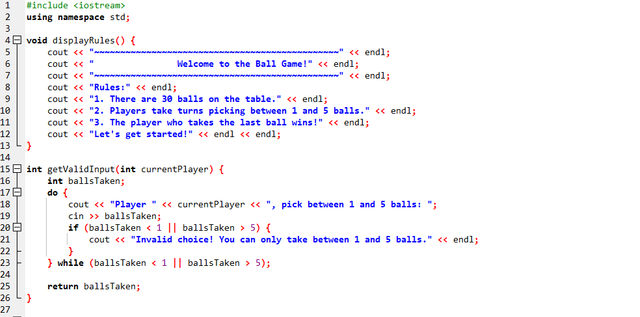
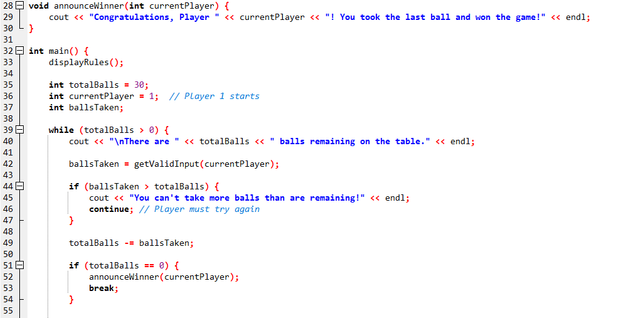

Explanation:
It is designed as a game in which two players will participate. First, the first player will start and when any player chooses his number, it is a condition for him to select the number from one to five. If the player selects another number, that input will be invalidated.
The game has a rule whereby no player can take more than the ball on the table.
When one player's turn is complete, he/she will be switched and the other player's turn will be played.
In this game, the player who picks up the last ball wins.
The game ends when there are no balls left on the table.
Here is the Output On Playing With Rules:
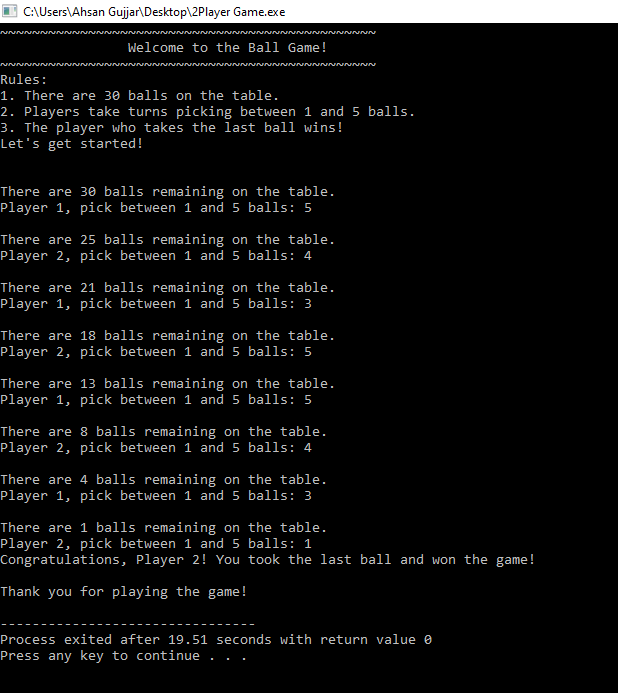
Here is the Output On Entering Wrong Key:
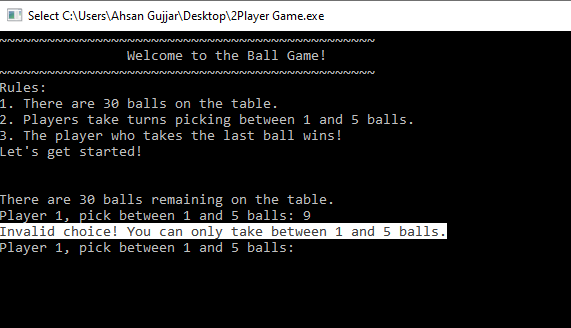
Here is the C++ Program Code:
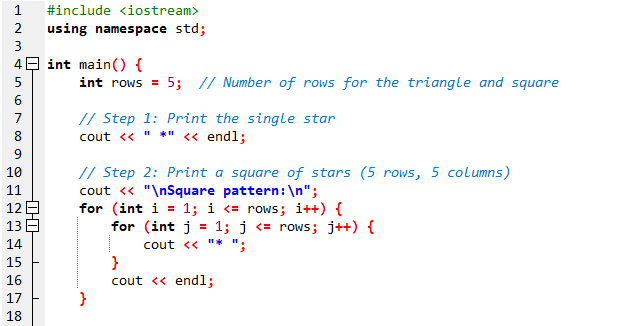
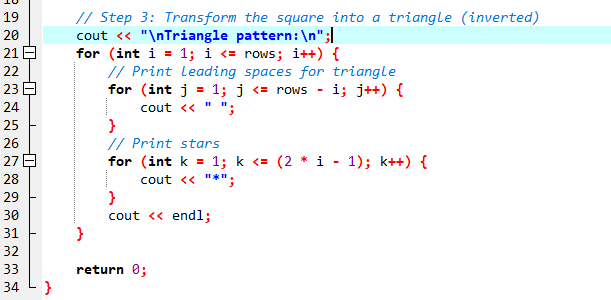
Explanation:
I have chosen the last triangle given in the post in this question and will construct it.
To complete this task, I will start with a star, first I will print a star, then I will print squares of stars, and then in the final form, I will print my triangle.
Our first step in the code above is to print a star in the center. That's all it does.
In the next second step, we will print a square of stars. In which we will have columns and rows and in this case they are five-cross-five.
The next step is our final step in which we will turn our stars square into a triangle.
Here is the Output
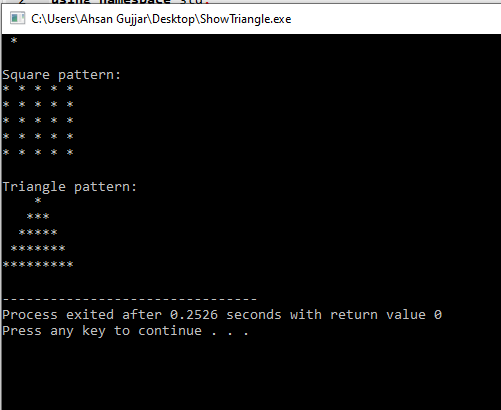
Thank you so much for staying here. I would like to invite @kouba01, @bossj23, and @ruthjoe to join this task.
Cc:
@sergeyk
Thank you so much dear @ruthjoe for your kind support and love. I wish you more success.