SEC-S20W6 Practice cycles and Guess the number game
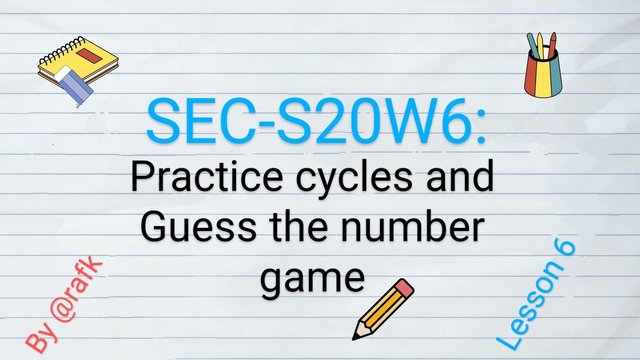
Practice cycles and Guess the number game
Hi everyone!
Finally, we are on week 6 of the steeemit engagement challenge and so far we have learned a lot about the basics of programming, not actually the full context, but at least we have the basis to get us started on our journey on programming. Without hesitation, I bring to you my last entry for this week's engagement challenge.
Experiment with colors—are there more than the ones we’ve used? How can you check?
Colors involve formatting text to look to find in appearance. There are several colors which we can used in our programming projects. This range from a variety such as red, blue, green, Magenta, Violet, Etc. just to name a few.
Colors can be brought about in our programming using Esc
sequence console To change the colors in our text or background. The escape are used to change the appearance of text in our program. In CPP We use the Escape sequence such as \033
or \e
Which is a synonym to the ASCII code for escape symbol. We can make the text for the edited as such as applying the board underline structure is in special characters as we see below.
Using the escape sequence, syntax We can be able to attribute other special combination Such as bold, italics or underline. This is possible by attributing [ ] After our escape code and then define in the parameters which we want to implement. For instance. \e[4:32m
print Out and underline text in green.
Color Theory Application.
To begin with, there are four main categories which we will be looking in this context.
- Standard color code and their representation.
- Background color code and their representation
- Special attribute color code and their representation
- Text styling color code and its application
Standard color code.
This refers to direct application of colors to our characters. Here are some of the color code and their colors.
N° | color code | Text color representation |
---|---|---|
1 | 30m | Black 🖤 |
2 | 31m | Red ♥️ |
3 | 32m | Green 💚 |
4 | 33m | Yellow 💛 |
5 | 34m | Blue 💙 |
6 | 35m | Magenta ♑ |
7 | 36m | Cyan 🩵 |
8 | 37m | White 🤍 |
• | • | |
• | 0m | Reset all attributes |
The above standard colors can be applied using the escape sequence As follows\033[30m or \e[30m , \033[31m..... For 🖤, ♥️
and so on.
Background color code
N° | color code | Background color representation |
---|---|---|
1 | 40m | Black background ⬛ |
2 | 41m | Red Background 🟥 |
3 | 42m | Green Background🟩 |
4 | 43m | Yellow Background🟨 |
5 | 44m | Blue Background🟦 |
6 | 45m | Magenta Background♑ |
7 | 46m | Cyan Background🩵 |
8 | 47m | White Background⬜ |
Here's the representation of how this background can be implemented in our code. \033[40m. , \033[41m for ⬛,🟥
and so on.
special attribute to text.
In this section, we can either make our text. Our background look high contrast., high brightness, and so on.
N° | color code | High brightness text representation |
---|---|---|
1 | 90m | Black ⬛ |
2 | 91m | Red 🟥 |
3 | 92m | Green 🟩 |
4 | 93m | Yellow 🟨 |
5 | 94m | Blue 🟦 |
6 | 95m | Magenta ♑ |
7 | 96m | Cyan 🩵 |
8 | 97m | White ⬜ |
N° | color code | High brightness Background color representation |
---|---|---|
1 | 100m | Black background ⬛ |
2 | 101m | Red Background 🟥 |
3 | 102m | Green Background🟩 |
4 | 103m | Yellow Background🟨 |
5 | 104m | Blue Background🟦 |
6 | 105m | Magenta Background♑ |
7 | 106m | Cyan Background🩵 |
8 | 107m | White Background⬜ |
Text styling
This is the future I representation of our text. We may like our text To look bold, underlined ..... And so on. This is possible with the following codes.
N° | color code | Background color representation |
---|---|---|
1 | 1m | Bold |
2 | 4m | underline |
3 | 5m | Blink |
4 | 7m | inverted color |
5 | 9m | strikethrough text |
After learning all the various color codes and background, let's see how this can be implemented in our program.
\e[1;31;47m
Is interpreted as bold red text in a white background.
print( "\033[4;33:47mWellcome to lesson 6 \033[0m" )
// print , "Welcome to lesson 6"
using Underline green text In a white background
Write My own version of the game "Guess the Number"—this time, creativity is more important than efficiency
In order to apply my knowledge and looks and cycle, I decided to write a number game which will select random numbers between 0 and 99. The name of my game is The checker
. With the checker, a user is a random number based on the difficulty levels. The user can choose; easy, normal Or difficult. Each level has a maximum number of attempt based on the option selected. Below is the code to the program.
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <string>
using namespace std;
int main() {
string name;
int number, guess, difficulty, max_number, attempts, max_attempts;
// Seed the random number generator
srand(time(0));
// Welcome message
cout << "\033[32mWelcome to Checker!\033[0m" << endl;
// Ask for the user's name
cout << "\033[34mWhat is your name? \033[0m";
cin >> name;
cout << "\033[32mHello, " << name << "!\033[0m" << endl;
// Choose difficulty level
cout << "\033[34mChoose a difficulty level (1: Easy, 2: Normal, 3: Difficult): \033[0m";
cin >> difficulty;
switch (difficulty) {
case 1:
max_number = 20;
max_attempts = 5;
break;
case 2:
max_number = 50;
max_attempts = 7;
break;
case 3:
max_number = 100;
max_attempts = 10;
break;
default:
cout << "\033[31mInvalid difficulty level. Defaulting to Normal.\033[0m" << endl;
max_number = 50;
max_attempts = 7;
break;
}
// Generate a random number based on difficulty
number = rand() % max_number;
// Main game loop
for (attempts = 0; attempts < max_attempts; attempts++) {
cout << "\033[34mGuess a number between 0 and " << max_number << ": \033[0m";
cin >> guess;
if (guess == number) {
cout << "\033[32mCongratulations, " << name << "! You guessed the number in " << attempts + 1 << " attempts!\033[0m" << endl;
return 0;
} else if (guess < number) {
cout << "\033[31mToo low!\033[0m" << endl;
} else {
cout << "\033[31mToo high!\033[0m" << endl;
}
}
cout << "\033[31mSorry, " << name << ". You ran out of attempts. The number was " << number << "\033[0m" << endl;
return 0;
}
Now let's see how the program works . Below is the screenshot of a played session based on difficulty level.
Note: The options initially are supposed to be in blue and a response in your Green, but based on the editor we could not have the desired results. ""chill"". Still not a problem.
Easy mode. I Got it!!👍.
Explanation.
The program begins By Brandon in the user I wake up message. And then proceed by wishing him good luck in his attempts.
Next day program ask the user to input their name. After which the user have inputted the names, a program proceeds by requesting the lever of difficulty the users want to play. They are very difficulty levels in this game.
The most important aspect of this game is that it is able to direct players by giving them hint to the right answer
. Each option selected is display a hint whether the number is too high or too low.
With this option, players can work toward a fixed direction depending on the hint.
We have easy
which involve selecting random numbers from 0 to 20
. The player has a maximum of attempt five attempts, after which the user is given the correct answer to the guess.
The next level is the normal mode,
which is selecting random numbers between 0 to 50
, here the player has a maximum of seven attempts. Once all these attempts have been exhausted, the program displays a message with the correct answer. If the player gets the right answer, the program will write a congratulation message. Congratulations!
In the difficulty mode
The player is requested to select random numbers from 0 to 100.
In this case there are a maximum of 10 attempt. If the player guesses the right answer, it displays a congratulation message, if not, it will say sorry you run out of attempt. The number ("number")
.
Make an Estimate of how long it will take us to guess a number if the range is from 0 to 1000 or from 0 to 10,000.
Estimating Guessing Time
- Problem Statement:
We're essentially trying to calculate the average number of guesses required to find a number within a given range using a guessing strategy.
Assumptions:
Random Number Generation: We'll assume the random number is generated uniformly within the specified range.
Optimal Guessing Strategy: We'll use a binary search algorithm, which is an efficient method for finding a target value in a sorted array. While our numbers aren't technically sorted, we can adapt the binary search principle.
Analysis:
Binary Search: using a binary search, we divide the search space in half with each guess.
Worst-case scenario: The target number is the median of the range.
Best-case scenario: The first guess is correct.
Calculations.
Range of 0 to 1000:
- Number of divisions required: log2(1000) ≈ 9.97
- So, in the worst case, it would take around 10 guesses.
Range of 0 to 10,000:
- Number of divisions required: log2(10000) ≈ 13.29
- So, in the worst case, it would take around 14 guesses.
Using proportions, If for instance it takes 2 seconds to get one attempt, then from 0 to 1,000, it will take 2×10 = 20 seconds.
From 0 to 10,000, it's a maximum of 14 attempts which gives us a time as; 2×14 = 28 seconds.
Conclusion:
Using a binary search strategy, we can estimate that guessing a number between 0 and 1000 would take around 10 guesses on average and time required (10* time for one attempt (s)), and guessing a number between 0 and 10,000 would take around 14 guesses on average and time required (10* time for 1 attempt(s)).
Disclaimer: This is a rough estimate and the actual number of guesses could vary depending on the specific numbers generated and the guessing strategy used.
Write a two-player game. There are 30 balls on the table, and players take turns picking between 1 and 5 balls. The winner is the one who takes the last ball. There's no need to develop a winning strategy. Simply program your codes to follow the rules, make the players take turns, and announce the winner at completion.
#include <iostream>
using namespace std;
int main() {
int balls_remaining = 30;
int player_turn = 1;
int balls_taken;
while (balls_remaining > 0) {
cout << "Player " << player_turn << ", how many balls do you take (1-5): ";
cin >> balls_taken;
if (balls_taken < 1 || balls_taken > 5 || balls_taken > balls_remaining) {
cout << "Invalid input. Please enter a number between 1 and 5, and ensure there are enough balls remaining." << endl;
continue;
}
balls_remaining -= balls_taken;
cout << "Balls remaining: " << balls_remaining << endl;
player_turn = (player_turn == 1) ? 2 : 1;
}
cout << "Player " << player_turn << " wins!" << endl;
return 0;
}
The game logic:
Initializes the number of balls remaining, the current player's turn, and a variable to store the number of balls taken.
The game continues in a loop while there are still balls remaining.
It prompts the current player to enter the number of balls they want to take.
Validates the input to ensure it's within the allowed range and there are enough balls left.
If the input is valid, it updates the number of balls remaining and switches to the next player's turn.
The loop continues until there are no balls left.
Once the last ball is taken, the game ends, and the current player is declared the winner.
We can see that player 2 is the winner. Owing to the fact that , four ball left, And he takes all the balls. You know again, we make sure an invalid input was not included. Otherwise the game will skip the player and go to the next player.
Follow the same path from a single star to a square, then transform the square into a triangle. Pick any triangle. Experienced students should choose one with a higher number, as it's more challenging.
In this section, we want to create shapes using a simple *
.
We start by printing the * In simple forms and then transforming it to large complex shape using the nested loop to property to print rows and columns.
#include <iostream>
using namespace std;
int main() {
// 1. Single Star
cout << "Single star:" << endl;
cout << "*" << endl;
// 2. Transform into a Line
int n = 9; // Number of stars in the line (columns)
cout << "\nLine of stars:" << endl;
for (int i = 0; i < n; i++) {
cout << "*";
}
cout << endl;
// 3. Transform into a Rectangle (with n=9 columns and k=5 rows)
int k = 5; // Number of rows
cout << "\nRectangle of stars (9 columns and 5 rows):" << endl;
for (int i = 0; i < k; i++) {
for (int j = 0; j < n; j++) {
cout << "*";
}
cout << endl;
}
// 4. Right-angle Triangle (about the x-axis)
cout << "\nMirrored right-angled triangle (about the x-axis):" << endl;
for (int i = k; i >= 1; i--) { // Mirrored version, limited to k rows
for (int j = 0; j < i; j++) {
cout << "*";
}
cout << endl;
}
return 0;
}
This brings us to the end of my task of this lessons. It was a nice time for them and I learned a lot throughout the season. I want a thank Mr.@sergeyk for dedicating He's time to teach us the basics of programming.
In order to end on a good note, I would like to invite a following persons participate with me in this contest. @chant, @sinomnnwigwe, and @fombae.
Cc: @sergeyk
Best regards: @rafk
Upvoted! Thank you for supporting witness @jswit.
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia